In this post we elaborately study how to make an Arduino based PWM signal generator circuit, which can be set or adjusted with a potentiometer or a pot to any preferred duty cycle ratio.
By Ankit Negi
WHAT IS PWM?
pwm or pulse width modulation as the name itself suggest is modulation of width of the pulses i.e. how long the pulse is high or low in a given time period. This changes duty cycle of the pulse which eventually determines the average value of pulse as duty cycle is on time divided by total time period.
And frequency plays very important role in pwm, which must be high enough to generate stable output
Pwm is done for variety of purposes like driving a device that works on low voltage or for switching purposes like in SMPS.
PWM USING ARDUINO UNO
Pwm is also one of the factors that make arduino a simplest development board, as pwm can be done by adding just one line code to your program. Note that there are separate digital pins available on arduino UNO for pwm which means these pins can give pwm output.
There are total 6 pwm pins available on arduino UNO that are 3, 5, 6,9,10 and11 out of 14 digital pins. Note that number of pwm pins vary from one type of arduino board to another.
Now there are two ways in which pwm can be performed by arduino:
1. By directly assigning an analog value to the pwm pin between 0 and 255.
Since digital pins in arduino can provide maximum of 5v that means 0 analog value is equal to 0 volts and 255 is equivalent to 5 volts.
And to perform this you have to just add this code to your program:
analogWrite( PWM pin no, value between 0 to 255);
For example: analogWrite(10,64);// write 64 analog value to pwm pin no 10.
Now this means:: (5/255)*64 volts= 1.25volts i.e. 25% duty cycle.
2. By assigning value according to the input received from analog pins of arduino.
Input can be taken from components like an IR sensor or a potentiometer.
Note that arduino receive analog input in terms of value between 0 to 1023 which is equivalent to 0 to 5 volts. So to perform pwm on a pin you must convert this input value in equivalence to number between 0 to 255 and this is called mapping in arduino’s language.
There is a simple code for this:
y= map(x,0,1023:0,255);// where x is the input variable
After this you can perform pwm on a pin using:
analogWrite(PWM pin no,y);// write received mapped value to pin 10
PWM EXAMPLE:
We are going to learn both the technique with this example. For this you need:
1. A potentiometer
2. Two leds
3. Two 100 ohm resistors
Make connections as shown in circuit diagram:
CIRCUIT DIAGRAM:

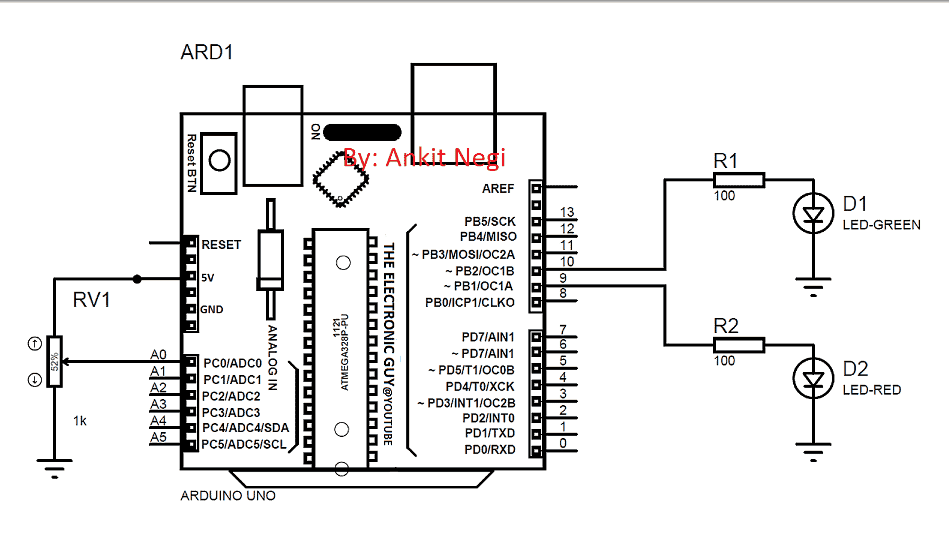
CODE:
int x;// initialise variables
int y;
void setup() {
pinMode(10,OUTPUT);//initialise pin 10 as output
pinMode(9,OUTPUT);//initialise pin 9 as output
pinMode(A0,INPUT);//initialise pin A0 as input from pot.
// put your setup code here, to run once:
}
void loop() {
analogWrite(9,125);// directly assigning value to pin 9 i.e. case1
x=analogRead(A0);// read values from potentiometer in terms of voltage
y= map(x,0,1023,0,255);// map those values from 0 to 255 // put your main code here, to run repeatedly:
analogWrite(10,y);// assigning value based on input from pot at pin A0 i.e. case 2
}
How it Works
The basic working of the proposed Arduino PWM signal generator project can be studied from the following paragraph
Pin no 9 can be assigned arbitrary pwm value whereas pin no. 10 gives pwm value in accordance to the position of the potentiometer with respect to ground. Keep changing this arbitrary value for pin 9 as well as rotate potentiometer to see different pwm output on both pins.