In this article I have explained how to make a simple 2-step Arduino programmable timer circuit, which can be used to switch an electrical load ON/OFF with independently adjustable ON and OFF timings.
For example if you want a light to remain ON for 24 hours and OFF for 2 hours, you can simply do this through a quick modification in the program code. In the same way you can customize the output timings to any other desired set of time intervals by changing the code appropriately.
You just have to compile and upload the following code to your Arduino board and start the timer function as per your specific application needs.
Program Code
void setup(){
pinMode(13, OUTPUT);
}
void loop(){
digitalWrite(13, HIGH);
delay(86400000);
digitalWrite(13, LOW);
delay(3600000);
}
In the above example code the lines delay(86400000); and delay(3600000); determine the output ON and OFF delay time intervals respectively, in milliseconds. Here, the figure 86400000 milliseconds corresponds to 24 hours, while 3600000 exhibits 1 hour delay.
You can customize these two values as per your personal preference to get the required output delays.
Once setup and powered, the Arduino will continue switching between the two step ON/OFF timing sequence. as long as power remains applied to the system.
Circuit Diagram
The complete circuit diagram along with the Arduino connections can be witnessed in the following diagram:

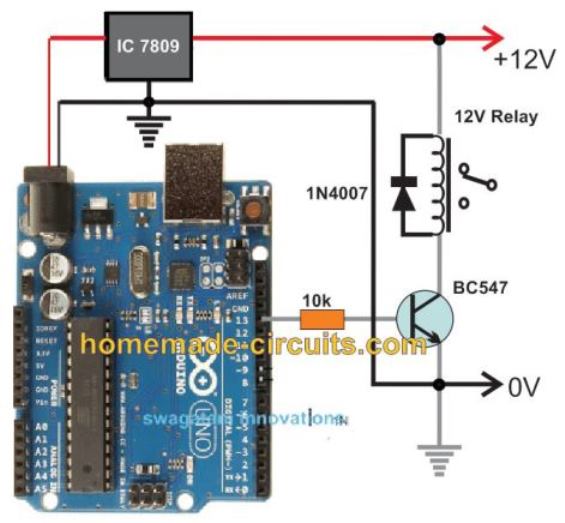
Arduino One-Shot Timer Circuit
If you don't want the timer to loop through the two step timer, instead want the timer to be a one-shot type, which will switch OFF permanently after the set delay, you can apply the following code:
int led = 13; // Pin 13 has an LED connected on most Arduino boards.
unsigned long DELAY_TIME = 10000; // 10 sec
unsigned long delayStart = 0; // the time the delay started
bool delayRunning = false; // true if still waiting for delay to finish
void setup() {
pinMode(led, OUTPUT); // initialize the digital pin as an output.
digitalWrite(led, HIGH); // turn led on
// start delay
delayStart = millis();
delayRunning = true;
}
void loop() {
// check if delay has timed out
if (delayRunning && ((millis() - delayStart) >= DELAY_TIME)) {
delayRunning = false; // finished delay -- single shot, once only
digitalWrite(led, LOW); // turn led off
}
}
If you want a discretely designed version of an identical programmable timer circuit, you can opt for this circuit
Parts Required for the Arduino Programmable Timer Circuit
- Arduino UNO Board = 1
- IC 7809 = 1
- BC547 = 1
- 1N4007 Diode = 1
- 10k 1/4 w resistor = 1
- Relay 12V/400 ohm/SPDT/5 amp = 1
- 12V AC to DC Adapter = 1
Can this be made to be rechargeable via usb? Are there a components available?
Your question is not clear, please elaborate…
Mr. swagatam, please if it is possible a timer circuit with repetition that works for example 3 seconds and stops for 2 minutes, then starts again with that time interval. I want the electronic circuit not to be with ardu
Hello Gelu, using the above Arduino method is much simpler than using discrete parts. If you do not want the Arduino, then you can use the concept explained in the following article:
Simple Programmable Timer Circuit
Tank You Mr.Swagatam
You are welcome!
Thank you for the information. If I wanted to have a 12v led turning off and on at different times for different durations, would it be possible using the code in the example? For example; on for 15 seconds off for 2 minutes, then on for 27 seconds and off for 5 minutes, on for 22 seconds and off for 4 minutes. Is a sequence like that possible?
No sorry, that may not be possible using this code, you can only select two adjustable timings with this code.
Could you please make me a sketch with these conditions: I have pins 3 & 11 open on a Arduino Uno. All others are used. I’m adding to a Sketch that controls other devices like a Stepper motor and a Servo. I want to: If I put a High into say pin 3, it starts a 0-5 second timer (at the same time I put the High in pin 3, I want it to Output a High on pin 11). After the timer has expired, the Output goes back Low. This in turn will bring the Input pin back low. Now the timer is ready for another Input, to repeat what just happened. I just want to control the Output to turn another circuit on/off. Is this possible? Thanks for any help.
Sorry, my Arduino knowledge is not good at all, so modifying or creating new programs can be very difficult for me.
Ok, thanks anyway. My coding isn’t good either! I’m just old and slow lol. I’ve been in electronics for 45 years, but due to personal reasons (I’ve got Tremors) it’s hard for me to do anymore. Anyway, thanks anyway.
Thank you Ralph, I appreciate your kind feedback. I hope you can still learn Arduino and fulfill your circuit requirements. All the best to you!
sir I did not found this arduino board please how can I try to another way to make some
You will have to use an Arduino UNO board for this which is easily available through any online store. Other alternative way is to use the following design:
Simple Programmable Timer Circuit
this circuit is difficulty found please my senior how to reduced some one is easy circuit micro controller
how to control after 2 minutes working also 118 minutes is off 2minutes on / 118 minutes is off
You will have to modify the code values for getting the desired ON/OFF timing.
Swagatam,
Thanks for posting this. I’d like to add a momentary button to start the timer cycle, how would this be done?
Thanks,
Todd
Hello Todd, I am not an Arduino expert, so it may be difficult for me to solve this query.
Thank You for most speedy reply- can I use a potentiometer for adjusting welding time- pause isn¨t’nt so crusial- welding in “spots” isnt always easy to control during the welding proces- maybe i can as experimetnally use a switch for differenting capasitors and a potmeter ,replacing R 2 – to a potentiometer some above the resistance for the fittet resistor- slope value- hoW many ohms for a middelvalue- about 5 sec??
You are welcome! Yes R2 can be replaced with a pot, for example a 500K pot, and C2 with a 220uF capacitor. Mke sure to put another fixed resistor such as 10K in series with the pot R2.
The circuit will function in the following manner: When power is switched ON, initially the relay will be OFF, and the LED will be OFF. After some delay the relay will switch ON, and then after some more delay the LED will switch ON and reset the relay to OFF, and the cycle will repeat.
I’m loving your site with nonsensfree smaal circuit , we all are able to complete. Maybe You can help me too-
I’m repairing my Welder , a nameless nearly 50 years old co2- welder, app. 250A. By mistake an bad luck i burned the motor for weldingmateriale- core,0,8 or 1mm thiknes- I have chosen to rebuild this velder, when a welder in same quality is pretty expencive- here in DK app 1000 us dollar, and i need a sort of a timer for
sequence velding, where is is velding for 5 sec and then pause for 5 sec- both period must be adjusteable from maybe 2-10 sec for the welding and maybe same for pause- it must drive a relay, which wil be the contact to the other circuit- and both periodes must be controlled with a potentiometer-hole controlling system is driven by 24 v DC- and of cource- as simpel as possible anit will be built on a trial bord vith velding -dots.
yours cincerrely Bjarne S. Nielsen
Thank you for liking this site! You can build and try the following transistorized two step timer circuit for your specific application:
R2 and C2 on both the stages can be adjusted for getting any desired timing for the relay ON/OFF sequencing
Добрый день. Можете помочь со скетчем таймера на ардуино или атини?
Таймер должен включать и выключать нагрузку на определенное время два раза в день.
Can I use arduino nano instead of arduino nano?
Yes you can use it…
Hello, sorry if this may seem off topick, am trying to make a inverter using arduino uno, am not good in cording so i generated a code from an android app, the inverter seem to be working ok, the only problem i have is how to give it feedback so the output voltage can be stable.
below is the generated code
#include
#define HO1 12
#define LO1 11
#define HO2 10
#define LO2 9
int f_sin=50;
int f_pwm=10000;
float sin_buffer[200];
float sampling_tot,pwm_period,sin_period,us=1000000;
int count=0,flag=0,Ampli=1000;
void setup() {
Serial.begin(9600);
pinMode(HO1,OUTPUT);
pinMode(LO1,OUTPUT);
pwm_period=(us*1)/f_pwm;
sin_period=(us*1)/f_sin;
sampling_tot=(sin_period/pwm_period)/2.0;
Serial.println(pwm_period);
Serial.println(sin_period);
Serial.println(sampling_tot);
for(int deg=0;deg(sampling_tot) && flag==1 ){
flag=0;
count=1;
TCCR1A=0b10110000;
}
if(count>(sampling_tot) && flag==0 ){
flag=1;
count=1;
TCCR1A=0b11100000;
}
count++;
if(flag==0){
Timer1.pwm(HO2,sin_buffer[count]* Ampli);
Timer1.pwm(LO2,sin_buffer[count]* Ampli);
digitalWrite(HO1,HIGH);
digitalWrite(LO1,LOW);
}
if(flag==1){
Timer1.pwm(HO2,sin_buffer[count]* Ampli);
Timer1.pwm(LO2,sin_buffer[count]* Ampli);
digitalWrite(HO1,LOW);
digitalWrite(LO1,HIGH);
}
}
Hello, if the inverter is working that means the code should be fine too…sorry, just like you I am too not good with coding stuff, so won’t be able to help you much in this regard.
Good day sir…happy Sunday…please I want be familiarize with arduino system..please help..how do I give a command it or program it? Thanks Engr.
Hello Sunshine, You can read the following article to get some basic tips regarding Arduino programming:
Learning Basic Arduino Programming – Tutorial for the Newcomers
Dear Sir;
I am in need of Arduino controlled reverse and forward relay controlled project details.
Following are the steps:-
1. After power ON, only after pushing ON push button (PB1), after 15 seconds, both relay1 (Motor1) and relay2 (Motor2) will start automatically simultaneously and stopped simultaneously, by pushing OFF push button (PB2) .
2. Relay3 (Motor3) will remain stopped, unless and until we press direction change push button (PB3).
3. When we will press PB3, relay2 stops and relay3 will ON after a timing gap of 15 seconds. Both relay2 and relay3 are interlocked. And stopped through PB2.
4. Along with PB3, there is also provision for a proximity switch (PB3 OR proximity switch) to change direction as per step number 3.
5. All three relay1, relay2 and relay3 will start with PB1 and stopped with PB2.
6. Relay1 is controlled with PB1 and PB2 only.
Thanks in advance for your kind guidance and support.
Sanjiv Mohbansi
sbmohbansi@gmail.com
Dear Sanjiv, I am not good with Arduino coding, so won’t be able to help you with this project.
Thank you Sir for your kind reply!!
Else as per your view, is there any option without using Arduino??
Awaiting your suggestion.
Thanks in advance!!
Yes it is possible using a simple flip flop circuit
Please Sir can you elaborate on how to load a bootloader to ATMEGA 328?
Thank you
Sorry Dan, I have no idea about it.
Thank you very much Sir for your quick responds. its exactly what I need.
You are welcome Dan!
wow Thanks a lot Swagatam the code (sketch) is working very well.
Glad it’s working Dan, hope you succeed with the project.