In this post I will show how to construct a digital ammeter using 16 x 2 LCD display and Arduino. We will understand the methodology of measuring current using a shunt resistor and implement a design based on Arduino. The proposed digital ammeter can measure current ranging from 0 to 2 Ampere (absolute maximum) with reasonable accuracy.
How Ammeters Work
There are two types of ammeters: Analog and digital, their workings are way different from each other. But, they both have one concept in common: A shunt resistor.
A shunt resistor is a resistor with very small resistance placed between the source and the load while measuring the current.
Let’s see how an analog ammeter works and then it will be easier to understand the digital one.

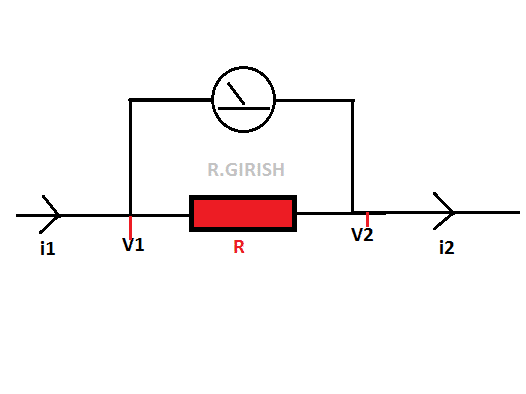
A shunt resistor with very low resistance R and assume some kind analog meter is connected across the resistor who’s deflection is directly proportional to voltage through the analog meter.
Now let’s pass some amount of current from left hand side. i1 is the current before entering the shunt resistor R and i2 will be the current after passing through shunt resistor.
The current i1 will be greater than i2 since it dropped a fraction of current through shunt resistor. The current difference between the shunt resistor develops very small amount of voltage at V1 and V2.
The amount of voltage will be measured by that analog meter.
The voltage developed across the shunt resistor depends on two factors: the current flowing through the shunt resistor and the value of the shunt resistor.
If the current flow is greater through the shunt the voltage developed is more. If the value of the shunt is high the voltage developed across the shunt is more.
The shunt resistor must be very tiny value and it must possess higher wattage rating.
A small value resistor ensures that the load is getting adequate amount of current and voltage for normal operation.
Also the shunt resistor must have higher wattage rating so that it can tolerate the higher temperature while measuring the current. Higher the current through the shunt more the heat is generated.
By now you would have got the basic idea, how an analog meter works. Now let’s move on to digital design.
By now we know that a resistor will produce a voltage if there is a current flow. From the diagram V1 and V2 are the points, where we take the voltage samples to the microcontroller.
Calculating Voltage to Current Conversion
Now let’s see the simple math, how can we convert the produced voltage to current.
The ohm’s law: I = V/R
We know the value of the shunt resistor R and it will be entered in the program.
The voltage produced across the shunt resistor is:
V = V1 – V2
Or
V = V2 – V1 (to avoid negative symbol while measuring and also negative symbol depend on direction of current flow)
So we can simplify the equation,
I = (V1 – V2)/R
Or
I = (V2 - V1)/R
One of the above equations will be entered in the code and we can find the current flow and will be displayed in the LCD.
Now let’s see how to choose the shunt resistor value.
The Arduino has built in 10 bit analog to digital converter (ADC). It can detect from 0 to 5V in 0 to 1024 steps or voltage levels.
So the resolution of this ADC will be 5/1024 = 0.00488 volt or 4.88 millivolt per step.
So 4.88 millivolt/2 mA (minimum resolution of ammeter) = 2.44 or 2.5 ohm resistor.
We can use four 10 ohm, 2 Watt resistor in parallel to get 2.5 ohm which was tested in the prototype.
So, how can we say the maximum measurable range of the proposed ammeter which is 2 Ampere.
The ADC can measure from 0 to 5 V only i.e. . Anything above will damage the ADC in the microcontroller.
From the tested prototype what we have observed that, at the two analog inputs from point V1 and V2; when the current measured value X mA, the analog voltage reads X/2 (in serial monitor).
Say for example, if the ammeter reads 500 mA the analog values on serial monitor reads 250 steps or voltage levels. The ADC can tolerate up to 1024 steps or 5 V maximum, So when the ammeter reads 2000 mA, the serial monitor reads 1000 steps approx. which is near to 1024.
Anything above 1024 voltage level will damage the ADC in Arduino. To avoid this just before 2000 mA a warning message will prompt on LCD saying to disconnect the circuit.
By now you would have understood how the proposed ammeter works.
Now let’s move on to constructional details.
Schematic diagram:
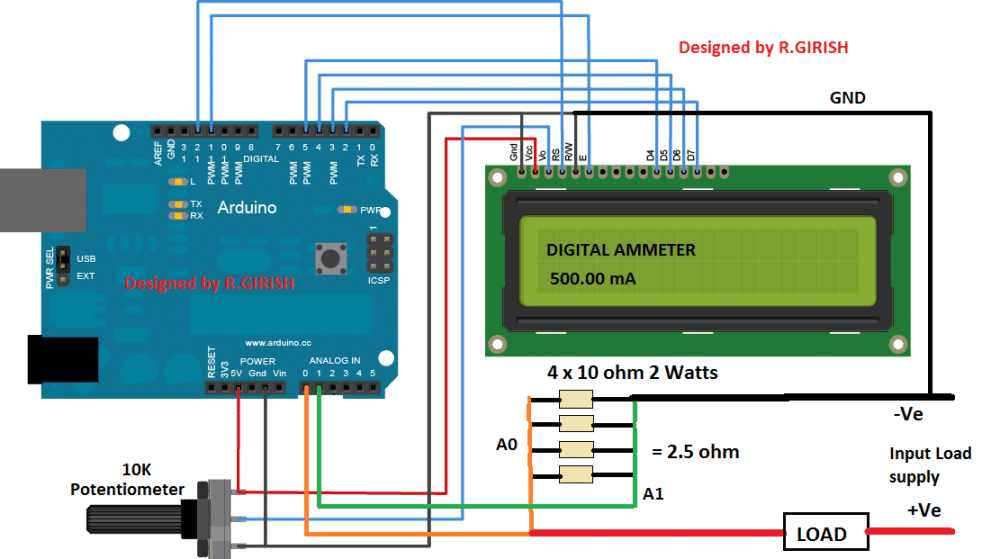
The proposed circuit is very simple and beginner friendly. Construct as per the circuit diagram. Adjust the 10K potentiometer to adjust display contrast.
You can power the Arduino from USB or via DC jack with 9 V batteries. Four 2 watt resistors will dissipate the heat evenly than using one 2.5 ohm resistor with 8- 10 watt resistor.
When no current is passing the display may read some small random value which you may ignore it, this might be due to stray voltage across measuring terminals.
NOTE: Don’t reverse the input load supply polarity.
Program Code:
//------------------Program Developed by R.GIRISH------------------//
#include <LiquidCrystal.h>
#define input_1 A0
#define input_2 A1
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
int AnalogValue = 0;
int PeakVoltage = 0;
float AverageVoltage = 0;
float input_A0 = 0;
float input_A1 = 0;
float output = 0;
float Resolution = 0.00488;
unsigned long sample = 0;
int threshold = 1000;
void setup()
{
lcd.begin(16,2);
Serial.begin(9600);
}
void loop()
{
PeakVoltage = 0;
for(sample = 0; sample < 5000; sample ++)
{
AnalogValue = analogRead(input_1);
if(PeakVoltage < AnalogValue)
{
PeakVoltage = AnalogValue;
}
else
{
delayMicroseconds(10);
}
}
input_A0 = PeakVoltage * Resolution;
PeakVoltage = 0;
for(sample = 0; sample < 5000; sample ++)
{
AnalogValue = analogRead(input_2);
if(PeakVoltage < AnalogValue)
{
PeakVoltage = AnalogValue;
}
else
{
delayMicroseconds(10);
}
}
input_A1 = PeakVoltage * Resolution;
output = (input_A0 - input_A1) * 100;
output = output * 4;
while(analogRead(input_A0) >= threshold)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Reached Maximum");
lcd.setCursor(0,1);
lcd.print("Limit!!!");
delay(1000);
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Disconnect now!!");
delay(1000);
}
while(analogRead(input_A0) >= threshold)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Reached Maximum");
lcd.setCursor(0,1);
lcd.print("Limit!!!");
delay(1000);
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Disconnect now!!");
delay(1000);
}
lcd.clear();
lcd.setCursor(0,0);
lcd.print("DIGITAL AMMETER");
lcd.setCursor(0,1);
lcd.print(output);
lcd.print(" mA");
Serial.print("Volatge Level at A0 = ");
Serial.println(analogRead(input_A0));
Serial.print("Volatge Level at A1 = ");
Serial.println(analogRead(input_A1));
Serial.println("------------------------------");
delay(1000);
}
//------------------Program Developed by R.GIRISH------------------//
If you have any specific question regarding this Arduino based digital ammeter circuit project, please express in the comment section, you may receive a quick reply.
Hi Swag,
i try to build this Circuit and upload the Code to Arduino Uno but aim getting following fonts only on my lcd
ññññññññññññññññ
ññññññññññññññññ
and aim using LCD keyboard Shield on arduino uno. (arduinolearning.com/wp-content/uploads/2014/12/lcd-keypad-shield.jpg)
Dear bro,
Please help me to build 4 channel voltmeter using aurdrino uno board with LCD 16 x2 ( direct measurement auto ranging 0 to 50v DC without any selector switch I.e 0-5v and 5-50v )
Thank you
(Deepak)
Hi Deepak, the idea is already present in many online websites, please search “4 channel Arduino voltmeter” you will be able to find quite a few of them.
This is an amazing tutorial. Is there a way I could measure smaller currents; in the micro-Ampere range?
Hi Nicole,
Thanks,
No you can’t measures in micro-amps with this circuit because arduino has limited ADC resolution.
There could be external modules which can help you measure in micro amps.
Regards
There are no branches for the current in the shunt circuit shown. There is only one current which is flowing through the shunt resistor. Hence, I1 and I2 are same. Please correct the posting. Anyway, the current flowing through the shunt is V1-V2/R where R is shunt resistor. The arduino sketch is correct.
The posting is probably written by considering the fact that if the current is checked across I1 and ground with an ammeter and then across I2 and ground we will find a difference in current where I2 reading will be less than I1.
I2 = V /shunt R
Thanks for your articles. I enjoy them very much. I have one small correction for this one, however. Kirchhoff’s current law (KCL) tells us that I1 must equal I2.
Regards,
Thanks Richard, however I am sorry it seems you have misinterpreted the law, according to Kirchoff’s current law the algebraic sum of the currents at a junction point in a circuit network is always zero…therefore i1 and i2 will be definitely different but there algebraic sum will be zero satisfying the law…I hope it makes sense!