In this post we will be learning how to interface Nokia 5110 display with arduino microcontroller and how to display some text, we will also be constructing a simple digital clock and finally we will be exploring graphical capabilities of the Nokia 5110 display.
By
Nokia was the most popular mobile phone brand across the globe before they jump into smartphone market. Nokia was known for manufacturing robust phones and one of the iconic and most robust of all was Nokia 3310.
Nokia brand made lot of noise across social media and meme started floating around internet and most of the meme was about 3310 model, due to its great durability with hard-core users. Some legit source says Nokia phones even saved some people’s life from bullets.
After the reduction in demand for these models in the market there were lot of displays left unused. Now they are refurbished and launched in market for our customized needs.
If want one for hands-on, you no need to create a mini-nuclear explosion around your area to salvage one from your old Nokia phone 🙂 It is commonly available in E-commerce sites.
Illustration of Nokia 5110 display:
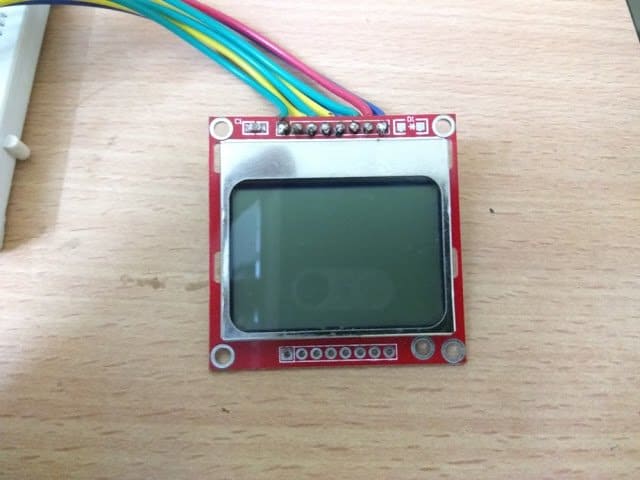
Fun fact: Nokia 5110 display was also used in 3310 model and some more other Nokia phone models too.
Now let’s see how to connect the display with arduino.
Connect Display with Arduino
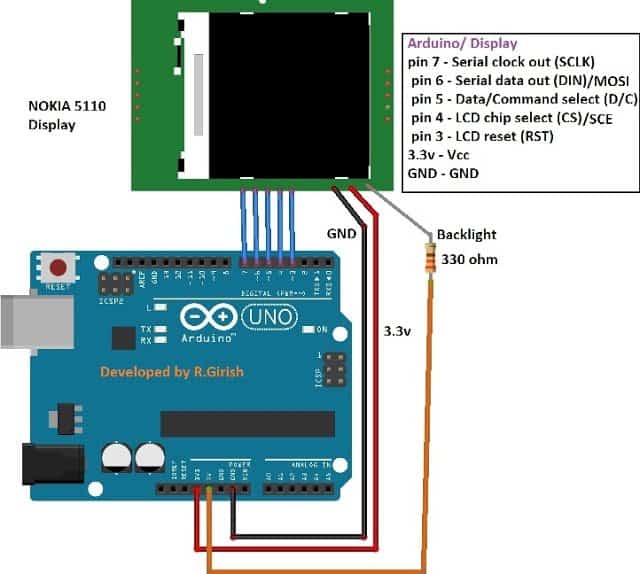
The display is monochrome and it has 84x48 pixels which can display text and even graphics.
The display consists of 8 pins: Vcc, GND, reset, chip select (CS), command select, serial data out, Serial clock and backlight.
The display is designed to work at 3.3V and applying 5V will damage the display, so care must be taken while handling it.
The display has backlight functionality which is commonly in white or blue in colour. 5V is given to backlight with 330 ohm current limiting resistor.
Pins 7, 6, 5, 4 and 3 are connected to the digital pins of the display. It is not mandatory to know how the arduino communicates with display in order to use it; we will add appropriate library files to the arduino software which will take care of the communication between arduino and display.
Now let’s display some text.
Displaying Text
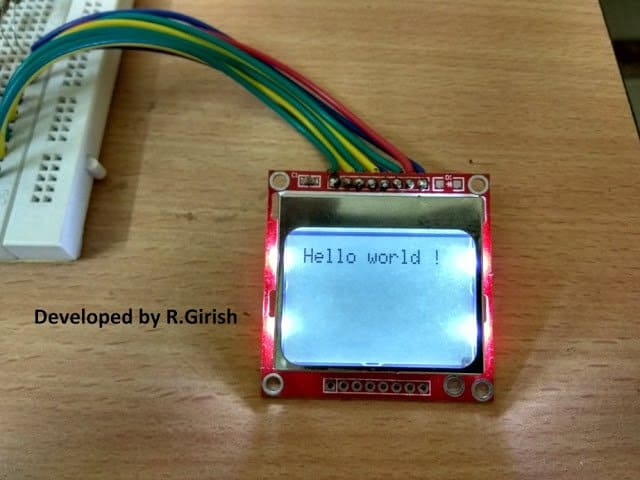
Before you upload the code you must download the library files and add to your arduino IDE.
• github.com/adafruit/Adafruit-PCD8544-Nokia-5110-LCD-library
• github.com/adafruit/Adafruit-GFX-Library
Program for Hello world:
//------------Program Developed by R.Girish--------//
#include <SPI.h>
#include <Adafruit_GFX.h>
#include <Adafruit_PCD8544.h>
Adafruit_PCD8544 display = Adafruit_PCD8544(7, 6, 5, 4, 3);
void setup()
{
display.begin();
display.setContrast(50);
display.clearDisplay();
}
void loop()
{
display.setTextSize(1);
display.setTextColor(BLACK);
display.print("Hello world !");
display.display();
delay(10);
display.clearDisplay();
}
//------------Program Developed by R.Girish--------//
If you want to explore more about the coding part, you may take a look at example program, which showcased about graphics, text colour (black/white), test size, text rotation and much more.
Now let’s construct a digital clock.
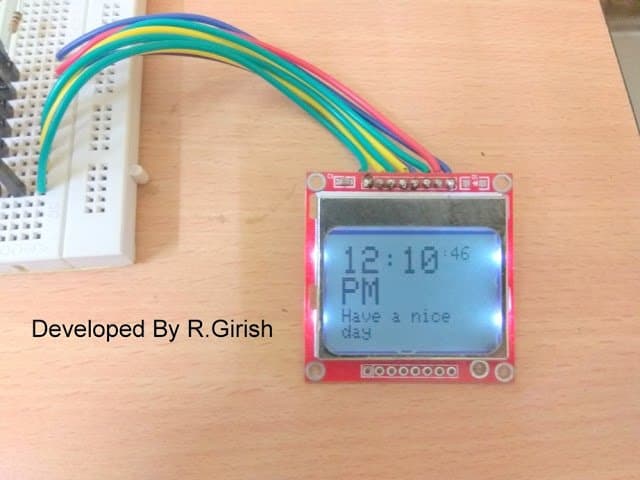
Circuit diagram for Digital clock:
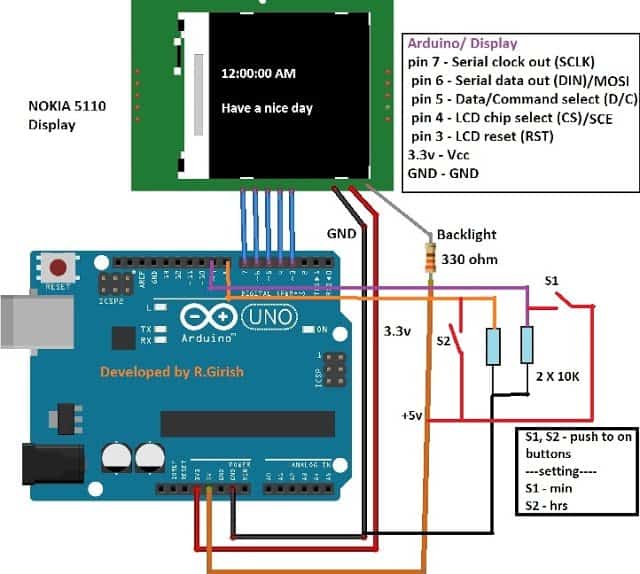
The schematic is same as previous one, only the difference is the two 10K ohm pull-down resistors for setting time are connect to pin #8 and pin # 9; rest of the circuit is self-explanatory.
Program for Digital clock:
//----------------Program developed by R.Girish-------//
#include <SPI.h>
#include <Adafruit_GFX.h>
#include <Adafruit_PCD8544.h>
Adafruit_PCD8544 display = Adafruit_PCD8544(7, 6, 5, 4, 3);
int h=12;
int m;
int s;
int flag;
int TIME;
const int hs=8;
const int ms=9;
int state1;
int state2;
void setup()
{
display.begin();
display.setContrast(50);
display.clearDisplay();
}
void loop()
{
s=s+1;
display.clearDisplay();
display.setTextSize(2);
display.print(h);
display.print(":");
display.print(m);
display.setTextSize(1);
display.print(":");
display.print(s);
display.setTextSize(2);
display.setCursor(0,16);
if(flag<12) display.println("AM");
if(flag==12) display.println("PM");
if(flag>12) display.println("PM");
if(flag==24) flag=0;
display.setTextSize(1);
display.setCursor(0,32);
display.print("Have a nice day");
display.display();
delay(1000);
if(s==60)
{
s=0;
m=m+1;
}
if(m==60)
{
m=0;
h=h+1;
flag=flag+1;
}
if(h==13)
{
h=1;
}
//-----------Time setting----------//
state1=digitalRead(hs);
if(state1==1)
{
h=h+1;
flag=flag+1;
if(flag<12) display.print(" AM");
if(flag==12) display.print(" PM");
if(flag>12) display.print(" PM");
if(flag==24) flag=0;
if(h==13) h=1;
}
state2=digitalRead(ms);
if(state2==1)
{
s=0;
m=m+1;
}
}
//-------- Program developed by R.GIRISH-------//
Now, let’s discuss graphical capabilities of the display. The Nokia 5110 display has 84x48 pixels, which can show very limited graphics that too in monochrome. It may not be as capable colour displays in smartphones but, it is very useful if we need to display logos or symbol in your project.
Illustration of graphics using Nokia 5110 display:
Popular troll face:
Dr. A.P.J Abdul Kalam:
As we can see that using a monochrome display, still we are able display some photos or logos directly from arduino. We no need any kind of external memory such as SD card.
The process of converting a photo into “C” code is subject of another article, where we will be illustrating step by step process.
If you have any queries, please express through the comment section.