In this article I have explained, how to send and receive SMS using GSM modem which is controlled by Arduino. Let us see, what GSM modem is, how to interface it with Arduino, how to send SMS with the setup.
We are also going to explore what are all applications we can achieve with GSM modem other than sending text message by a human.
What is GSM modem?
GSM stands for Global System for Mobile communications; it is a standard which was developed by ETSI (European Telecommunications Standard Institute) who described the protocols for 2G communication.
It is the first digital protocol for mobile communication which is optimized for full duplex voice communication. In a nutshell full duplex communication means both the parties can send/receive data (or voice) simultaneously.
The GSM protocol also allows transfer of packet data, such as GPRS and EDGE.
SIM800 GSM modem:

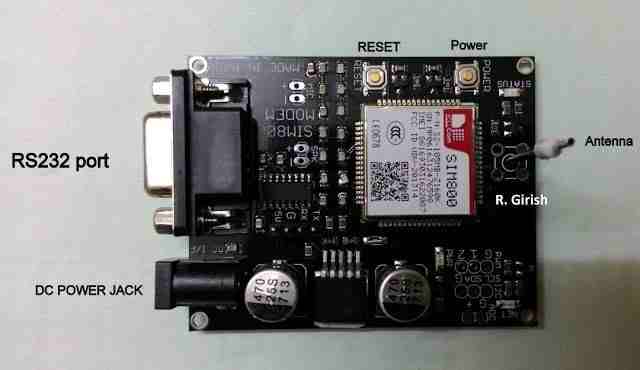
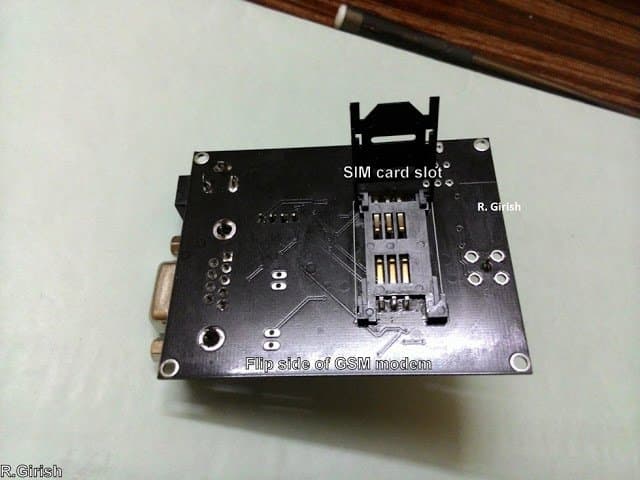
GSM modem is a hardware which accepts a valid SIM card (Subscriber Identity Module), basically any SIM will work, which supports GSM protocol and with a network subscription.
It is like a mobile phone without Screen and keypad. It has four I/O pins depending on the model you choose.
Two for TX and RX (transmit and receive), another two pins for VCC and GND, which is common in all.
It also consist of RS232 port for serial communication between the modem and computer, however we are not going to use in this project.
It has standard DC power jack, which can be powered from external power sources such as voltage adapters.
It has working voltage ranging from 5 to 12V on DC jack, depending on the model. It has 3 LED indicators, for power, status, and network.
The power LED indicates the presence of power, status LED indicates whether the GSM modem is operating or not, the Network LED indicates the establishment of mobile network.
Initially network LED blinks every one second while searching for network, once it establishes the mobile network it blinks every 3 seconds.
You need to press power button for 2 to 3 seconds for activating the GSM modem, once you done, it latch to the mobile network.
To verify that your GSM modem works, just call the number of which you have inserted the SIM card. You should get ring back tone. If it does, then your module is working fine.
We are going to use SIM800 GSM modem which supports quad-band 850/900/1800/1900 MHz. if you own a SIM900 modem, need not to worry, the program and circuit is compatible in this project.
Now, you would have gained some idea about GSM modem, now let’s learn how to interface it with arduino.
Circuit Diagram:
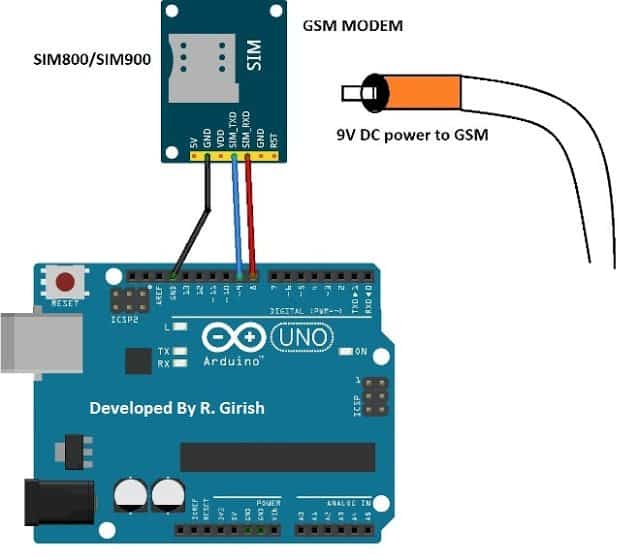
As you can infer form the diagram, the circuit connection is dead easy. You just need 3 male to female header pins. A USB cable is mandatory in this project, as we are going to communicate via serial monitor.
Always, power the GSM modem with external adaptor. The power from arduino is insufficient for the GSM modem; it could even overload the voltage regulator of the arduino.
That’s all about hardware part. Now, let’s move to coding.
Program:
//-------------Program developed by R.Girish---------------//
#include <SoftwareSerial.h>
#define rxPin 9 // gsm TX------> arduino 9
#define txPin 8 //gsm RX--------> arduino 8
SoftwareSerial mySerial = SoftwareSerial(rxPin, txPin);
char text[150];
String message="";
int x;
void setup()
{
Serial.begin(9600);
while (!Serial){}
mySerial.begin(9600);
delay(1000);
Serial.println("Write your message (with dot at end):");
}
void loop()
{
x=0;
while( Serial.available()>0 )
{
text[x] = Serial.read();
message += text[x];
x++;
if (text[x-1]==46)
{
Serial.println("Your message is sending......");
SendTextMessage();
ShowSerialData();
delay(1000);
Serial.println("r");
Serial.println("Success");
message="";
x=0;
}}}
void SendTextMessage()
{
mySerial.print("AT+CMGF=1r");
delay(1000);
mySerial.print("AT+CMGS="+91xxxxxxxxxx"r"); // Replace x with your 10 digit phone number
delay(1000);
mySerial.println(message);
mySerial.print("r");
delay(1000);
mySerial.println((char)26);
mySerial.println();
}
void ShowSerialData()
{
while(mySerial.available()!=0)
Serial.write(mySerial.read());
}
//-------------Program developed by R.Girish---------------//
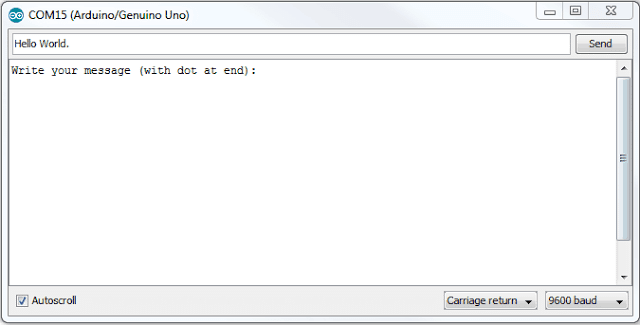
Don’t forget the dot (.) at every end of the message, otherwise it won’t sent the message to prescribed number in the program. Replace x with your 10 digital phone number in the program. Make sure you have a working SMS plan on your SIM card.
If you are not from India, please change the country code in the program.
For example:
For UK: +44
For US: +1
For Canada: +1
For Russia: +7
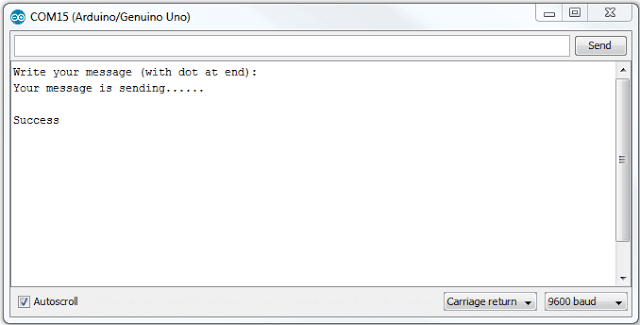
You can also automate the message which is sent by GSM modem by coding Arduino appropriately. You can receive automated message alerts on your phone such as: anti-theft alert, fire alarm alert, weather alert on your local area etc.
You can even connect to internet with GPRS in GSM modem, but it is subject of another article.
In one of the forth coming articles I have explained How to Receive SMS Using GSM Modem and Arduino
If you have further questions regarding how to send SMS using GSM Modem , feel free to ask in the comment section.
How to Receive SMS Using GSM Modem
In the above discussion I have explained how to send a text message using GSM modem and also discussed the basics the GSM modem.
In this section I will elucidate regarding how to receive SMS via serial monitor of the arduino IDE. We are not only going to receive SMS but, also send text message by pressing different keys. For an instant, pressing “s” will send pre-enter text message, pressing “r” will receive real time SMS.
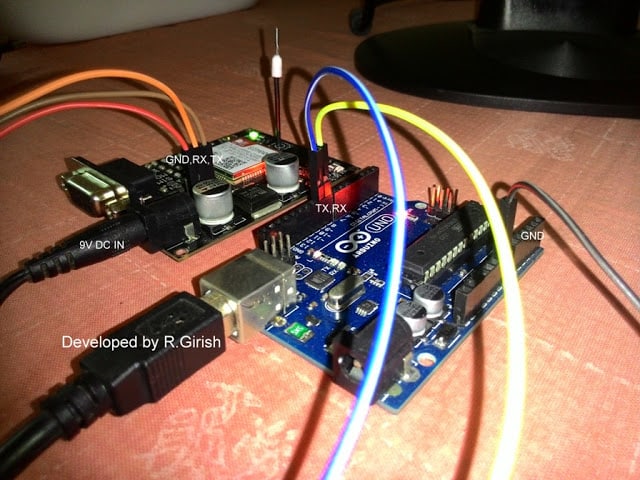
How it Works
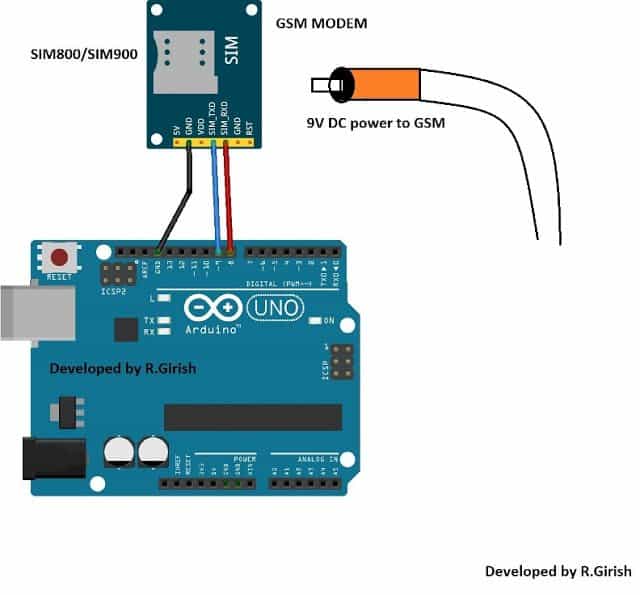
The circuit for receiving SMS using a GSM moden is very simple, you just need 3 male to female header pins. The TX of GSM modem is connected to pin #9 of arduino and RX of GSM modem is connected to pin #8 of arduino and the ground to ground connection is also given between GSM and arduino.
Always use external power supply for GSM modem, don’t connect 5Vcc from arduino to GSM modem, as there is good chance of overloading the voltage regulator of arduino.
Don’t forget to implement SMS rate cutter or something similar on your SMS subscription for reduction on your SMS expenses.
Otherwise you will end up empty account balance after sending several SMS, since there won’t be any acknowledgement from your cellular provider after every sent SMS, as the SIM card is in the GSM modem.
The only acknowledgement you get is warning SMS, regarding your empty account, so be cautious about you expenses. Now let’s move to coding part this project.
Program:
//-----------------Program developed by R.Girish-------------//
#include <SoftwareSerial.h>
SoftwareSerial gsm(9,8);
void setup()
{
gsm.begin(9600); // Setting the baud rate of GSM Module
Serial.begin(9600); // Setting the baud rate of Serial Monitor (Arduino)
delay(100);
}
void loop()
{
if (Serial.available()>0)
switch(Serial.read())
{
case 's':
Send();
break;
case 'r':
Recieve();
break;
case 'S':
Send();
break;
case 'R':
Recieve();
break;
}
if (gsm.available()>0)
Serial.write(gsm.read());
}
void Send()
{
gsm.println("AT+CMGF=1");
delay(1000);
gsm.println("AT+CMGS="+91xxxxxxxxxx"r"); // Replace x with mobile number
delay(1000);
gsm.println("Hello I am GSM modem!!!");// The SMS text you want to send
delay(100);
gsm.println((char)26); // ASCII code of CTRL+Z
delay(1000);
}
void Recieve()
{
gsm.println("AT+CNMI=2,2,0,0,0"); // AT Command to receive a live SMS
delay(1000);
}
//-----------------Program developed by R.Girish-------------//
Entering the Phone Numbers
Enter the recipient phone number on “xxxxxxxxxxx” in the program with your country code at the beginning.
Enter the text that you want to send in the program within the quotation mark: gsm.println("Hello I am GSM modem!!!"); // The SMS text you want to send
Compile the program and upload to arduino.
Insert the SIM card and power the GSM modem with external power supply and press the power button for 3 seconds (depending the model), wait for 10 to 20 seconds to establish mobile network, the network LED should blink once in every 3 seconds. If everything is stated above is done, we are ready to go for next step.
Now open serial monitor and press “r” the GSM modem is ready to receive SMS. Now send a text message from any mobile phone to number of the SIM which is inserted on GSM modem.
The text message should pop up on the serial monitor, something similar to illustrated below:
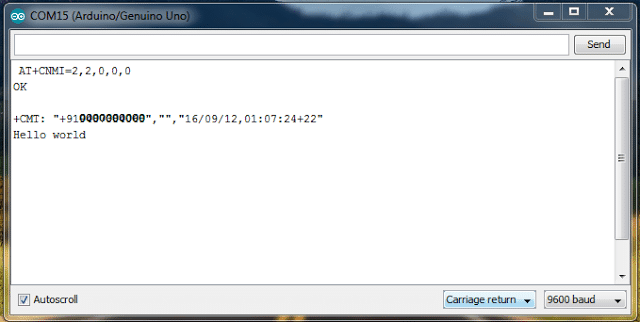
The “Hello world” is the message sent to GSM modem and the number from which the text message is sent also displayed.
Now, let send SMS to the pre-entered number in the program with pre-entered message. Press “s” and you will see something similar illustrated below: The sent SMS is “Hello I am GSM modem”.
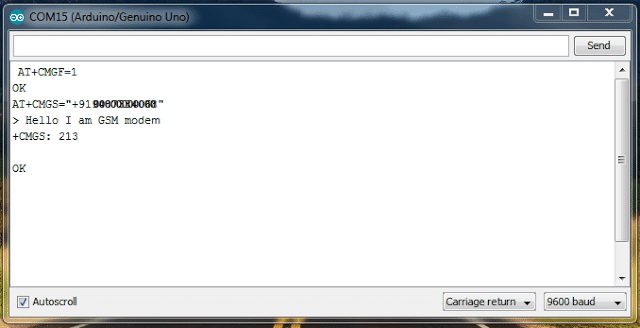
Now, you know how to send and how to receive SMS using GSM modem.
Have Questions? Please Leave a Comment. I have answered over 50,000. Kindly ensure the comments are related to the above topic.
hello sir,
i am not getting the reply from gsm module..can you lease help to how to get the reply..and there was a error showing in the program at in this line
mySerial.print(“AT+CMGS=”+91xxxxxxxxxx”r”);
if we given like shown below its not showing any error
mySerial.print(“AT+CMGS=\”+91xxxxxxxxxx\”\r”);