In this post I will show how to construct an incubator using Arduino which can self-regulate its temperature and humidity. This project was suggested by Mr. Imran yousaf who is an avid reader of this website.

Introduction
This project was designed as per the suggestions from Mr. Imran, but some additional modification is done to make this project universally suitable for all.
You may use your creativity and imagination to get this project done.
So let’s understand what an incubator is? (For noobs)
Incubator is an enclosed apparatus whose internal environmental is isolated from ambient environment.
This is to create favourable environment for the specimen under care. For example incubators are used to grow microbial organism in laboratories, incubators are used in hospitals to take care of prematurely born infants.
The kind of incubator we are going to build in this project is for hatching chicken eggs or any other bird eggs.
All incubators have one thing in common; it regulates the temperature, humidity and provides adequate oxygen supply.
You can set temperature and humidity by pressing the provided buttons and also it shows the internal temperature and humidity in real time. Once both parameters set it automatically controls the heating element (bulb) and vaporizer (humidifier) to meet the set point.
Now let’s understand the apparatus and design of the incubator.
The chassis of the incubator may be of Styrofoam / thermocol box or acrylic glass which can provide good thermal insulation. I would recommend Styrofoam / thermocol box which will be easier to work with.
Apparatus design:
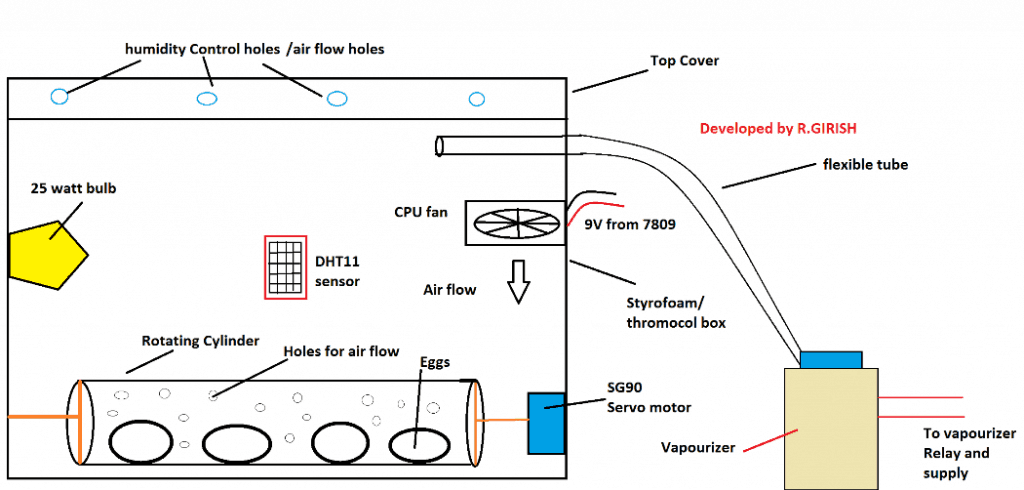
A 25 watt bulb acts as heat source; higher wattage may hurt the eggs in a small container. The humidity is provided by vaporizer, you may use the vaporizer something similar as shown below.
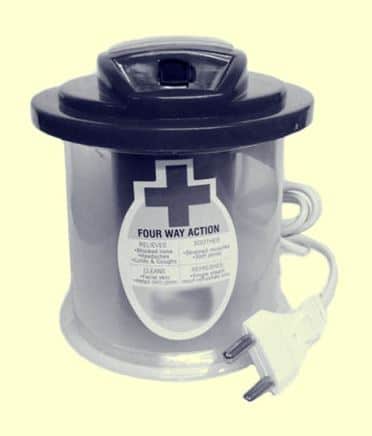
It produces thick stream of steam which will be inlet to incubator. The steam can be carried via any flexible tube.
The flexible tube can be something similar as shown below:
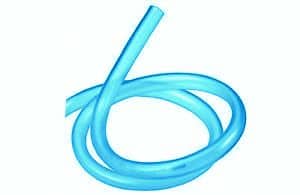
The steam may be inlet from top of the Styrofoam / thermocol box as shown in the apparatus design, so that excess heat will escape though the humidity control holes and less hurting the eggs.
There is a cylinder carrying eggs with several holes around it, connected to a servo motor. The servo motor rotates the cylinder 180 degree every 8 hours thus rotates the eggs.
The rotation of the eggs prevents the embryo sticking to the shell membrane and also provides contact with the food material in the egg, especially at early stage of incubation.
The rotating cylinder must have several numbers of holes so that proper air circulation will be present and also the cylinder must be hollow on both sides.
The rotating cylinder can be PVC tube or cardboard cylinder.
Paste an ice cream stick on both end of the hollow cylinder such that the ice cream stick makes two equal semi circles. Paste the arm of the servo motor at middle of the ice cream stick. On the other side poke a hole and paste a tooth pick firmly.
Insert the tooth pick inside box and paste the servo on opposite wall inside the box. The cylinder must stay horizontal as possible, now the cylinder can rotate as the servo motor rotates.
And yes, use your creativity to make the things better.
If you want to accommodate more eggs make more such cylinders and multiple servo motor can be connected on same control line pin.
The humidity control holes can be made by poking a pencil through the Styrofoam / thermocol box at the top. If you made lot of unnecessary holes or if humidity or temperature is escaping too fast you may cover some of the holes using electrical or duct tape.
The DHT11 sensor is heart of the project which may be placed at the middle of any four sides of incubator (inside) but away from the bulb or humidity inlet tube.
CPU fans can be placed as shown in the apparatus design for air circulation. For proper air circulation use at-least two fans pushing the air in opposite direction, for example: one of the CPU fan pushing downwards and another CPU fan pushing upwards.
Most CPU fan works on 12V but at 9V works just fine.
That’s all about the apparatus. Now let’s discuss on the circuit.
Schematic Diagarm:
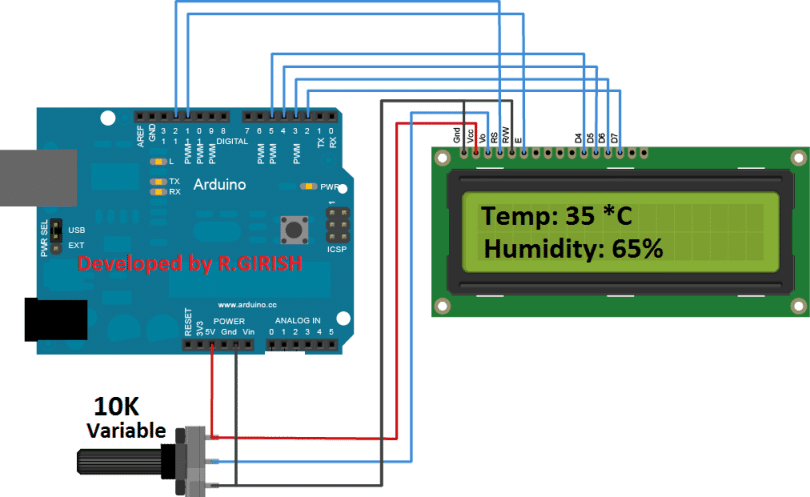
The above circuit is for Arduino to LCD connection. Adjust 10K potentiometer for adjusting LCD contrast.
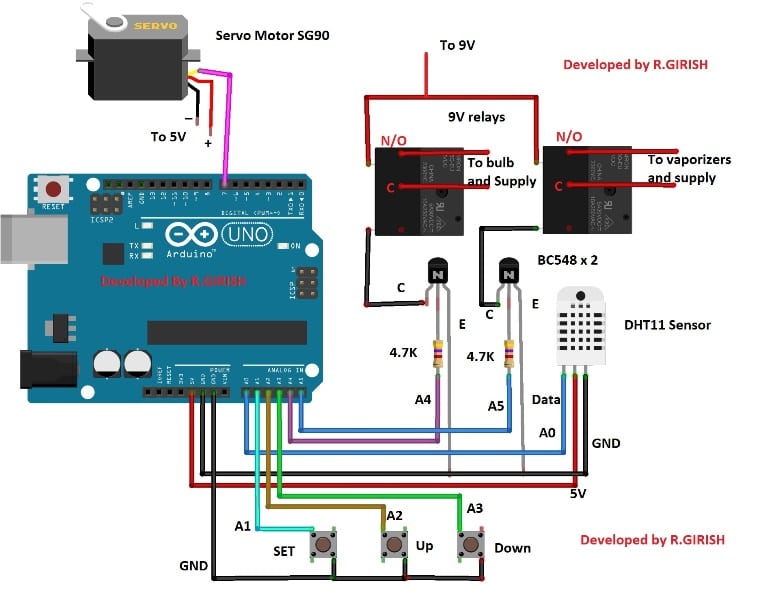
The Arduino is the brain of the project. There are 3 push buttons for setting temperature and humidity. The pin A5 controls the relay for vaporizer and A4 for the bulb. The DHT11 sensor is connected to pin A0. The pins A1, A2 and A3 used for push buttons.
The pin #7 (non-PWM pin) is connected to servo motor’s control wire; multiple servo motors can be connected to pin #7. There is misconception that servo motors works only with PWM pins of Arduino, which is not true. It works happily on non PWM pins too.
Connect a diode 1N4007 across the relay coil in reverse bias to eliminate high voltage spikes while switching on and off.
Power Supply:
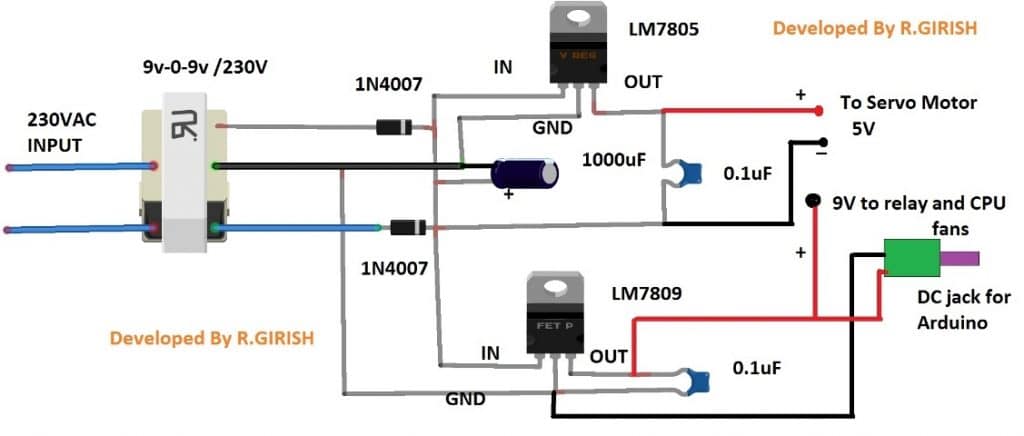
The above power supply can provide 9 V and 5 V supply for relay, Arduino, Servo motor (SG90) and CPU fans. The DC jack is provided for powering the Arduino.
Use heat sinks for the voltage regulators.
That concludes the power supply.
Download the library DHT sensor:
https://arduino-info.wikispaces.com/file/detail/DHT-lib.zip
Program Code:
//------------------Program Developed by R.GIRISH-------------------//
#include <LiquidCrystal.h>
#include <Servo.h>
#include <dht.h>
#define DHT11 A0
const int ok = A1;
const int UP = A2;
const int DOWN = A3;
const int bulb = A4;
const int vap = A5;
const int rs = 12;
const int en = 11;
const int d4 = 5;
const int d5 = 4;
const int d6 = 3;
const int d7 = 2;
int ack = 0;
int pos = 0;
int sec = 0;
int Min = 0;
int hrs = 0;
int T_threshold = 25;
int H_threshold = 35;
int SET = 0;
int Direction = 0;
boolean T_condition = true;
boolean H_condition = true;
LiquidCrystal lcd(rs, en, d4, d5, d6, d7);
Servo motor;
dht DHT;
void setup()
{
pinMode(ok, INPUT);
pinMode(UP, INPUT);
pinMode(DOWN, INPUT);
pinMode(bulb, OUTPUT);
pinMode(vap, OUTPUT);
digitalWrite(bulb, LOW);
digitalWrite(vap, LOW);
digitalWrite(ok, HIGH);
digitalWrite(UP, HIGH);
digitalWrite(DOWN, HIGH);
motor.attach(7);
motor.write(pos);
lcd.begin(16, 2);
Serial.begin(9600);
lcd.setCursor(5, 0);
lcd.print("Digital");
lcd.setCursor(4, 1);
lcd.print("Incubator");
delay(1500);
}
void loop()
{
if (SET == 0)
{
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Set Temperature:");
lcd.setCursor(0, 1);
lcd.print(T_threshold);
lcd.print(" *C");
while (T_condition)
{
if (digitalRead(UP) == LOW)
{
T_threshold = T_threshold + 1;
lcd.setCursor(0, 1);
lcd.print(T_threshold);
lcd.print(" *C");
delay(200);
}
if (digitalRead(DOWN) == LOW)
{
T_threshold = T_threshold - 1;
lcd.setCursor(0, 1);
lcd.print(T_threshold);
lcd.print(" *C");
delay(200);
}
if (digitalRead(ok) == LOW)
{
delay(200);
T_condition = false;
}
}
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Set Humidity:");
lcd.setCursor(0, 1);
lcd.print(H_threshold);
lcd.print("%");
delay(100);
while (H_condition)
{
if (digitalRead(UP) == LOW)
{
H_threshold = H_threshold + 1;
lcd.setCursor(0, 1);
lcd.print(H_threshold);
lcd.print("%");
delay(100);
}
if (digitalRead(DOWN) == LOW)
{
H_threshold = H_threshold - 1;
lcd.setCursor(0, 1);
lcd.print(H_threshold);
lcd.print("%");
delay(200);
}
if (digitalRead(ok) == LOW)
{
delay(100);
H_condition = false;
}
}
SET = 1;
}
ack = 0;
int chk = DHT.read11(DHT11);
switch (chk)
{
case DHTLIB_ERROR_CONNECT:
ack = 1;
break;
}
if (ack == 0)
{
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Temp:");
lcd.print(DHT.temperature);
lcd.setCursor(0, 1);
lcd.print("Humidity:");
lcd.print(DHT.humidity);
if (DHT.temperature >= T_threshold)
{
delay(3000);
if (DHT.temperature >= T_threshold)
{
digitalWrite(bulb, LOW);
}
}
if (DHT.humidity >= H_threshold)
{
delay(3000);
if (DHT.humidity >= H_threshold)
{
digitalWrite(vap, LOW);
}
}
if (DHT.temperature < T_threshold)
{
delay(3000);
if (DHT.temperature < T_threshold)
{
digitalWrite(bulb, HIGH);
}
}
if (DHT.humidity < H_threshold)
{
delay(3000);
if (DHT.humidity < H_threshold)
{
digitalWrite(vap, HIGH);
}
}
sec = sec + 1;
if (sec == 60)
{
sec = 0;
Min = Min + 1;
}
if (Min == 60)
{
Min = 0;
hrs = hrs + 1;
}
if (hrs == 8 && Min == 0 && sec == 0)
{
for (pos = 0; pos <= 180; pos += 1)
{
motor.write(pos);
delay(25);
}
}
if (hrs == 16 && Min == 0 && sec == 0)
{
hrs = 0;
for (pos = 180; pos >= 0; pos -= 1)
{
motor.write(pos);
delay(25);
}
}
}
if (ack == 1)
{
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("No Sensor data.");
lcd.setCursor(0, 1);
lcd.print("System Halted.");
digitalWrite(bulb, LOW);
digitalWrite(vap, LOW);
}
delay(1000);
}
//------------------Program Developed by R.GIRISH-------------------//
How to operate the Circuit:
· With completed hardware and apparatus setup, power the circuit ON.
· The display shows “set temperature” press up or down button to get the desire temperature and press “set button”.
· Now the display shows “set Humidity” press up or down buttons to get desire humidity and press “set button”.
· It begins the functioning of the incubator.
Please refer internet or get advice from a professional for temperature and humidity level for the eggs.
If you have any specific question regarding this Arduino automatic incubator temperature and humidity control circuit, feel free to express in the comment section. You may receive a quick reply.
Have Questions? Please Comment below to Solve your Queries! Comments must be Related to the above Topic!!