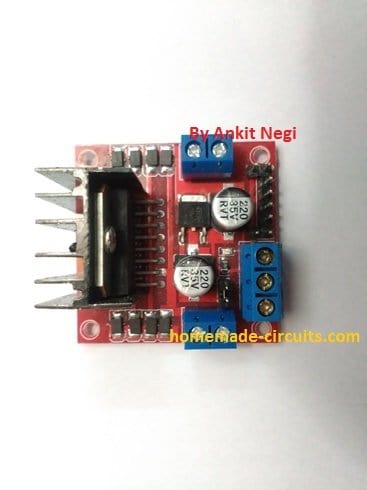
In this project we are going to control a manual robot through our cellphone using DTMF module and Arduino.
By: Ankit Negi, Kanishk Godiyal and Navneet Singh sajwan
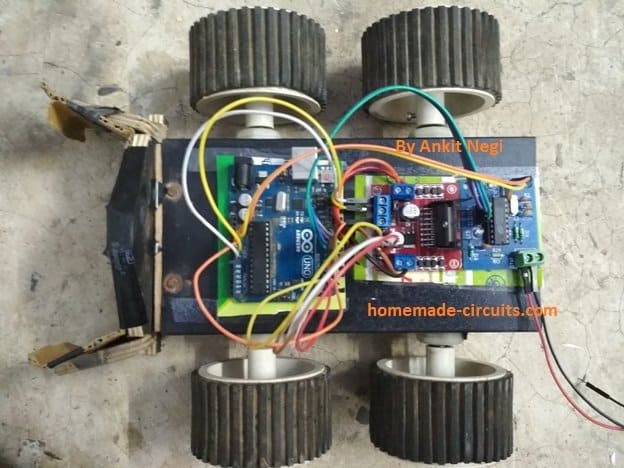
INTRODUCTION
In this project two cell phones, one for calling and one for receiving the call are used. The phone receiving the call is connected to the robot via audio jack.
The person who is calling can control the robot just by pressing the dial pad keys. (i.e. the robot can be operated from any corner of the world).
COMPONENTS REQUIRED
1 - Arduino UNO
2 – Manual robot
3 - 4 motors (here we used 300 r.p.m each)
4 - DTMF module
5 - Motor driver
6 – 12 volt Battery
7 - Switch
8 - Headphone Jack
9 – Two Cell Phones
10 – Connecting wires
ABOUT MANUAL ROBOT
A manual robot consists of chassis (body) in which three or four motors (which are screwed with tyres) can be attached depending on requirement.
Motors to be used depend on our requirement i.e. they can either provide high speed or high torque or a good combination of both. Applications like quad copter requires very high speed motors to lift against gravity while application like moving a mechanical arm or climbing a steep slope requires high torque motors.
Both motors on left and right side of robot are connected in parallel separately. Usually they are connected to a 12volt battery via DPDT(double pin double throw) switches.
But in this project we will use mobile phone instead of DPDTs to control the bot.
ABOUT MOTOR DRIVER
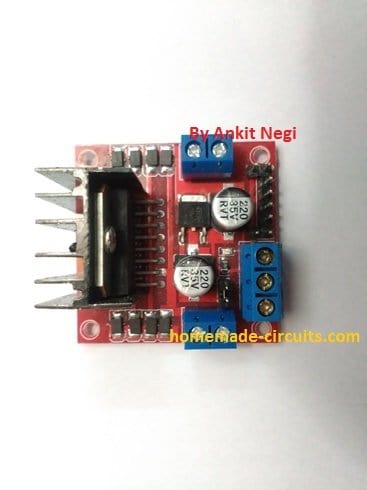
Arduino gives maximum current of 40mA using GPIO (general purpose input output) pins, while it gives 200mA using Vcc and ground.
Motors require large current to operate. We can’t use arduino directly to power our motors so we use a motor driver.
Motor driver contains H Bridge (which is a combination of transistors). The IC (L298) of motor driver is driven by 5v which is supplied by arduino.
To power the motors, it takes 12v input from arduino which is ultimately supplied by a 12 v battery. So the arduino just takes power from battery and gives to the motor driver.
It allows us to control the speed and direction of motors by giving maximum current of 2 amperes.
INTRODUCTION TO DTMF MODULE
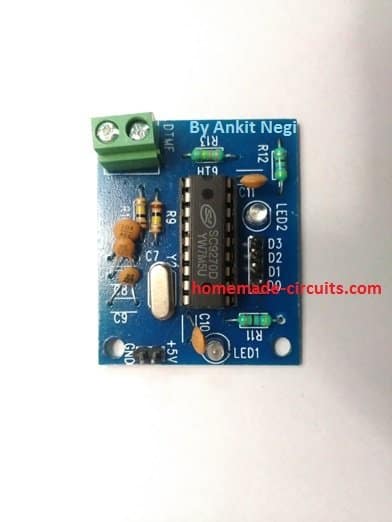
DTMF stands for Dual tone multi frequency. Our dial pad is a two toner multiple frequency i.e. one button gives a mixture of two tones having different frequency.
One tone is generated from a high frequency group of tones while other from a low frequency group. It is done so that any type of voice can’t imitate the tones.
So, it simply decodes the phone keypad’s input into four bit binary code. The frequencies of keypad numbers which we have used in our project is shown in the table below
DigitLow frequency(hertz)High frequency(hertz)2697133647701209677014778852133609411336
The binary decoded sequence of the dial pad’s digits is shown in the table below.
digitD3D2D1D010001200103001140100501016011070111810009100101010*1011#1100
CIRCUIT DIAGRAM
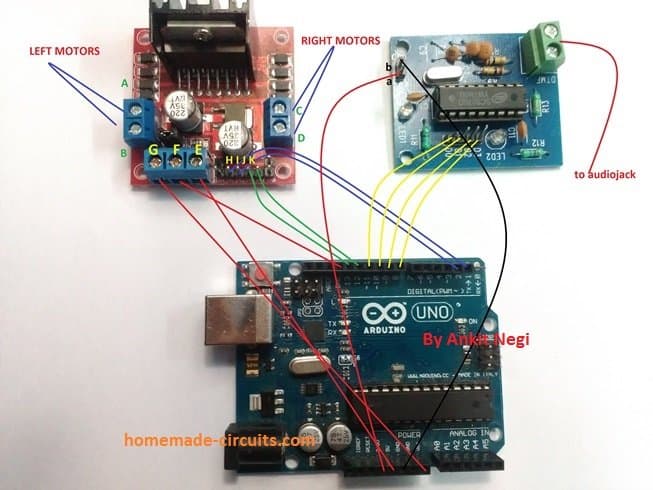
CONNECTIONS
Motor driver –
- Pin ‘A’ and ‘B’ controls left side motor while Pin ‘C’ and ‘D’ controls right side of motor. These four pins are connected to the four motors.
- Pin ‘E’ is to power IC(L298) which is taken from arduino (5v).
- pin ‘F’ is ground.
- Pin ‘G’ takes 12 volt power from battery via Vin pin of arduino.
- Pins ‘H’, ‘I’, ‘J’ and ‘K’ receives logic from arduino.
DTMF –
- pin ‘a’ is connected to 3.5 volt of arduino to power the IC (SC9270D).
- Pin ‘b’ is connected to ground.
- The input of DTMF is taken from phone via jack.
- The output in the form of binary data via (D0 – D3) pins goes to arduino.
ARDUINO –
- the output of DTMF from (D0 – D3) pins comes to digital pins of arduino. We can connect this output to any of the four digital pins varying from (2 – 13) in arduino. Here we used pins 8, 9, 10 and 11.
- Digital pins 2 and 3 of arduino are connected to pin number ‘H’ and ‘I’ of motor driver while pins 12 and 13 of arduino are connected to ‘J’ and ’K’.
- The arduino is connected to a 12 volt battery.
Program CODE-
int x ; // initialising variables
int y ;
int z ;
int w ;
int a=20 ;
void setup()
{
pinMode(2,OUTPUT) ; //left motor
pinMode(3,OUTPUT) ; //left
pinMode(8,INPUT) ; // output from DO pin of DTMF
pinMode(9,INPUT) ; //output from D1 pin of DTMF
pinMode(10,INPUT) ; //output from D2 pin of DTMF
pinMode(11,INPUT) ; // output from D3 pin of DTMF
pinMode(12,OUTPUT) ; //right motor
pinMode(13,OUTPUT) ; //right
Serial.begin(9600);// begin serial communication between arduino and laptop
}
void decoding()// decodes the 4 bit binary number into decimal number
{
if((x==0)&&(y==0)&&(z==0)&&(w==0))
{
a=0;
}
if((x==0)&&(y==0)&&(z==1)&&(w==0))
{
a=2;
}
if((x==0)&&(y==1)&&(z==0)&&(w==0))
{
a=4;
}
if((x==0)&&(y==1)&&(z==1)&&(w==0))
{
a=6;
}
if((x==1)&&(y==0)&&(z==0)&&(w==0))
{
a=8;
}
}
void printing()// prints the value received from input pins 8,9,10 and 11 respectively
{
Serial.print(" x ");
Serial.print( x );
Serial.print(" y ");
Serial.print( y );
Serial.print(" z ");
Serial.print( z );
Serial.print(" w ");
Serial.print( w );
Serial.print(" a ");
Serial.print(a);
Serial.println();
}
void move_forward()// both side tyres of bot moves forward
{
digitalWrite(2,HIGH);
digitalWrite(3,LOW);
digitalWrite(12,HIGH);
digitalWrite(13,LOW);
}
void move_backward()//both side tyres of bot moves backward
{
digitalWrite(3,HIGH);
digitalWrite(2,LOW);
digitalWrite(13,HIGH);
digitalWrite(12,LOW);
}
void move_left()// only left side tyres move forward
{
digitalWrite(2,HIGH);
digitalWrite(3,LOW);
digitalWrite(12,LOW);
digitalWrite(13,HIGH);
}
void move_right()//only right side tyres move forward
{
digitalWrite(2,LOW);
digitalWrite(3,HIGH);
digitalWrite(12,HIGH);
digitalWrite(13,LOW);
}
void halt()// all motor stops
{
digitalWrite(2,LOW);
digitalWrite(3,LOW);
digitalWrite(12,LOW);
digitalWrite(13,LOW);
}
void reading()// take readings from input pins that are connected to DTMF D0, D1, D2 and D3 PINS.
{
x=digitalRead(8);
y=digitalRead(9);
z=digitalRead(10);
w=digitalRead(11);
}
void loop()
{
reading();
decoding();
if((x==0)&&(y==0)&&(z==1)&&(w==0))
{
move_forward();
reading();
decoding();
printing();
}
if((x==1)&&(y==0)&&(z==0)&&(w==0))
{
move_backward();
reading();
decoding();
printing();
}
if((x==0)&&(y==1)&&(z==0)&&(w==0))
{
move_left();
reading();
decoding();
printing();
}
if((x==0)&&(y==1)&&(z==1)&&(w==0))
{
move_right();
reading();
decoding();
printing();
}
if((x==0)&&(y==0)&&(z==0)&&(w==0))
{
halt();
reading();
decoding();
printing();
}
a=20;
printing();
}
CODE EXPLANATION
- First of all, we initialise all variables before void setup.
- In void setup, all pins to be used are assigned as input or output according to their purpose.
- A new function “void decoding()” is made. In this function all the binary input that we get from DTMF is decoded to decimal by arduino. And variable assigned for this decimal value is a.
- Another function “void printing()” is made. This function is used to print input values from DTMF pins.
- Similarly, five functions are required functions are required to perform the required task. These functions are:
void move_left(); // robot turns left
void move_right() ; // robot turns right
void move_forward(); // robot moves forward
void move_backward() ; // robot moves backward
void halt() ; // robot stops
- Now these functions are used in void loop function to do their task whenever they are called according to input from dialpad of cellphone.
For example:::
if((x==0)&&(y==0)&&(z==1)&&(w==0))
{
move_forward();
reading();
decoding();
printing();
}
hence when button 2 is pressed or 0010 is received on input pins, arduino decodes this and thus these functions do their work : move_forward();
reading();
decoding();
printing();
CIRCUIT WORKING
The controls which we have used in our project are as follows –
2 – To move forward
4 – To turn left
6 – To turn right
8 – To move backwards
0 – to stop
After making a call to the phone connected to the robot, the person opens his dial pad.
- If ‘2’ is pressed. The DTMF receives the input, decodes it in its binary equivalent number i.e. ‘0010’ and sends it to digital pins of arduino. The arduino then sends this code to the motor driver as we have programmed when the code will be ’0010’, the motors will rotate in clockwise direction and hence our robot will move forward.
- If ‘4’ is pressed then its equivalent code is ‘0100’ and according to the programming the left side motors will stop and only right side motors will rotate clockwise and hence our robot will turn left.
- If ‘6’ is pressed then the right side motor will stop and only left side motors will rotate clockwise and hence our robot will turn right.
- If ‘8’ is pressed then our motors will rotate in anticlockwise direction and thus our robot will move backward.
- If ‘0’ is pressed then all our motors will stop and robot will not move.
In this project we have assigned a function to five dial pad numbers only. We can add any type of other mechanism and assign a dial pad number to that mechanism to make an upgraded version of this project.
POINTS TO KEEP IN MIND
1 – The jack should not be loose.
2 – Phone keypad tones should be maximum.
3 – Internet/ Wi-Fi of receiving phone should be closed to avoid interference effects.
4 – Left pin (i.e. pin ‘b’) of DTMF is ground and right pin (i.e. pin ‘a’) is connected to 3.3v.
Prototype images of the cellphone controlled robot car circuit using DTMF
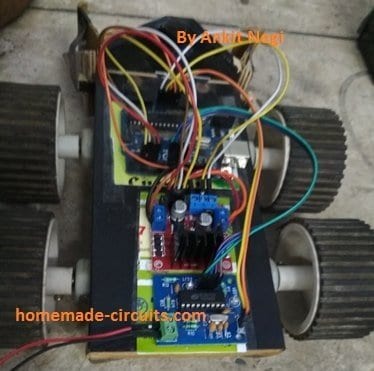
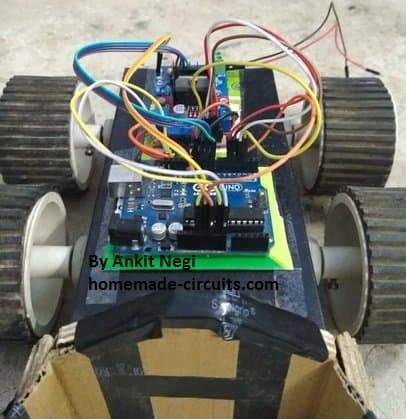
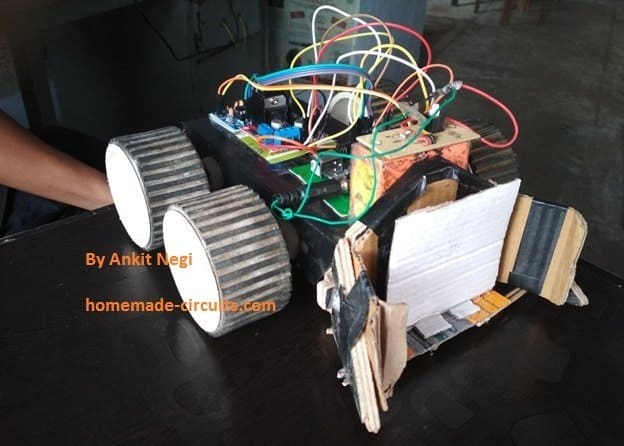
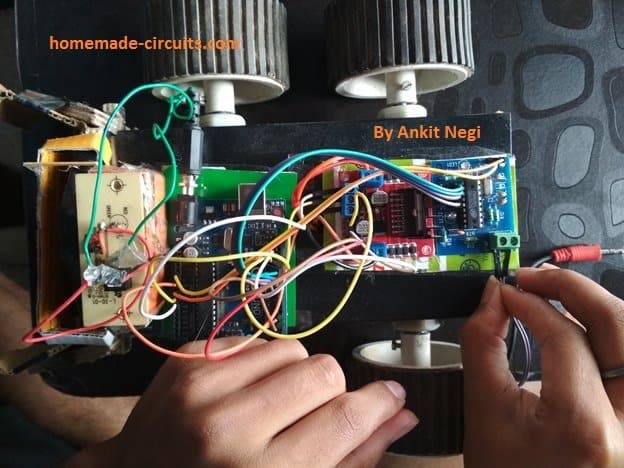
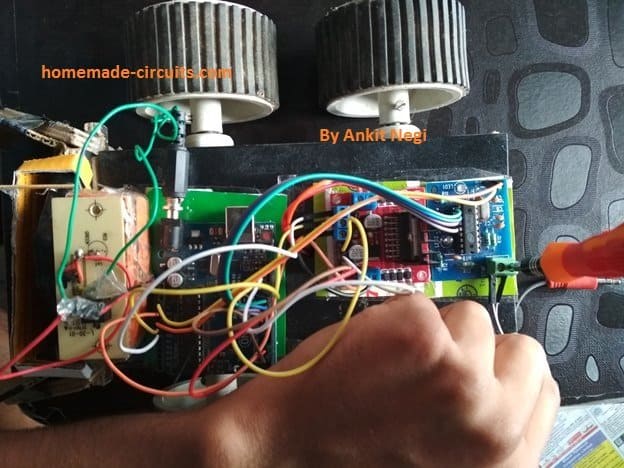
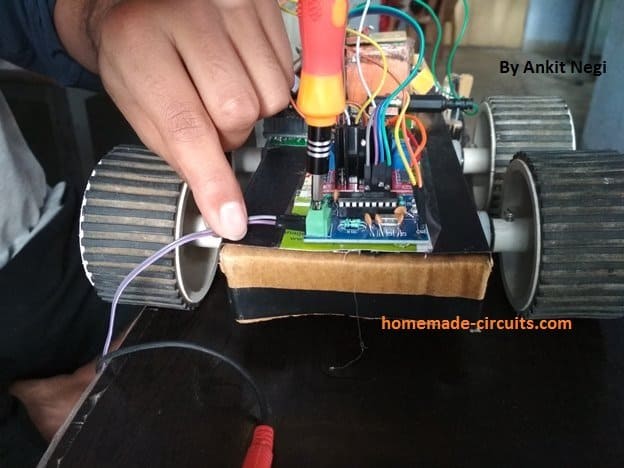
With over 50,000 comments answered so far, this is the only electronics website dedicated to solving all your circuit-related problems. If you’re stuck on a circuit, please leave your question in the comment box, and I will try to solve it ASAP!