In this post I will show how to construct a password based mains ON/OFF switch circuit, which can turn ON and OFF the AC mains supply, only when correct password is entered. This prevents the danger of electrocution of the technician who might be currently working with the high voltage lines and equipment.

Password Protection for Utility Mains Lines is Crucial
The biggest nightmare for any electrician or technician is an accidental activation of AC lines by someone, which might kill or cause fatal damage to the body organs in a blink of an eye.
This password protected mains ON/OFF switch circuit prevents such unfortunate incident and allows the technician to turn ON the AC mains supply safely by entering correct password and not just by flipping a lever.
This project presents the facility to change password which is stored in the EEPROM of Arduino’s microcontroller.
Human body or even animal body has its own electrical system, which helps to send information from one part of the body to another part. The information is send as electrical signals which have measurable amplitude and frequency. It also helps to contract and relax the muscles, for example our heart.
Fun Fact: Heart has a multivibrator like signal generator called “SA node” or “Sinoatrial”; which controls the heart rate. If Sinoatrial fails we have to apply external electrical signal to heart using pacemaker.
Any surge to existing electrical signals of our body will make lose control over our own body parts. That’s why people feel getting stuck and paralyzed when they come in contact with an open electrical LIVE wire.
Our body have reasonable resistance and also good amount of electrical conductance. We know that any element with resistance generate heat when electric current is passed.
This applies to human body too; the heat damages the organs and may cause blood to boil. Soon or later the person may die if he/she got electrocuted long enough.
That’s enough medical electronics for now. Let’s move on to technical details.
The project consists of LCD display, a 4 x 3 alphanumeric keypad, a status LED and relay.
Schematic for Arduino and LCD connection:
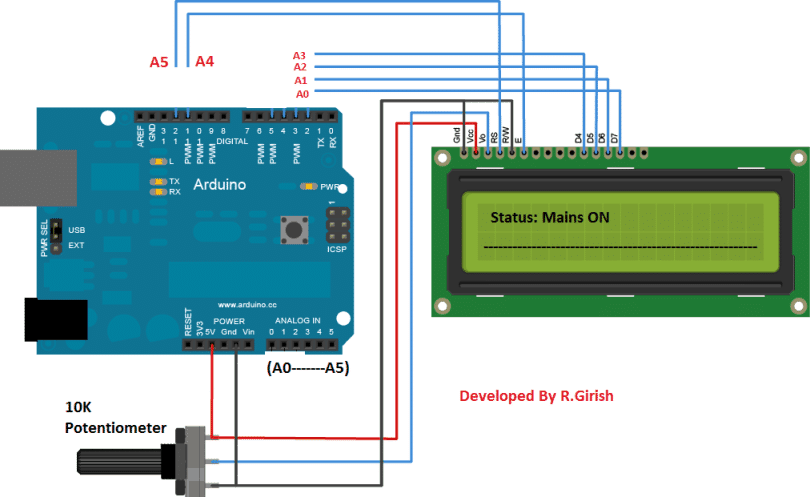
The display is connected to analog pins of the arduino from A0 to A5. The display is unusually connected to analog pins (which functions same as connected to digital pins) so that keypad can be connected to digital pins (from 2 to 9).
Adjust display contrast using the 10 K ohm potentiometer.
Keypad connection:
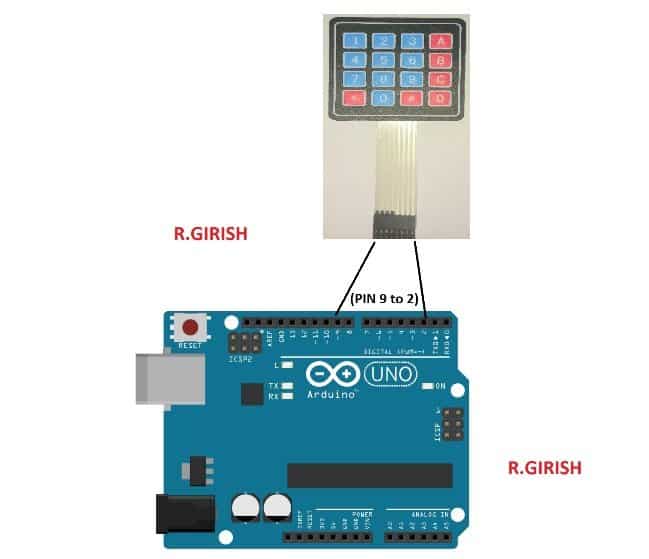
The keypad has 8 cables which should be connected to Arduino, from pin #2 to pin #9. The left most wire of keypad must go to pin #9 and connect the succeeding right to next wire of keypad to pin# 8, 7 ,6, 5, 4, 3, 2, the last or the right most wire of keypad must go to pin #2.
Rest of the electrical connections:
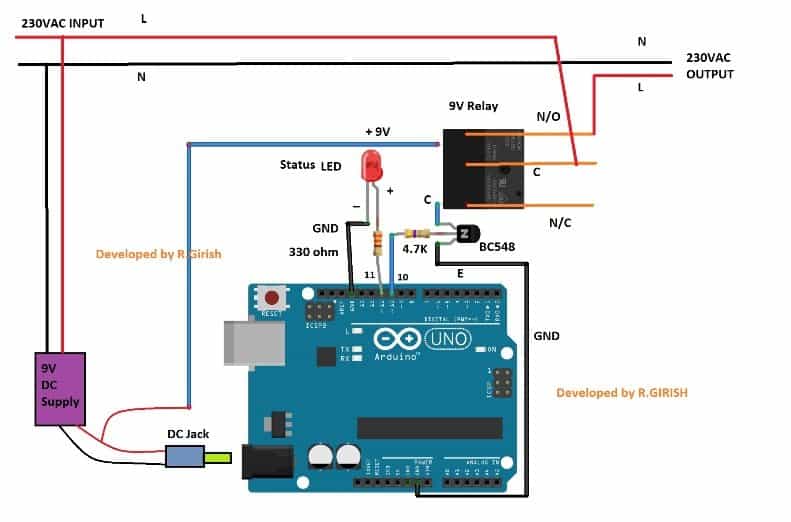
You need to download and add the keypad library from the following link: github.com/Chris--A/Keypad before compiling the code.
EEPROM of Arduino microcontroller would have some random values stored initially. We have to reset to zero, so that our main program don’t get confused. To rest EEPROM values to zero upload the below program first and then upload the main program second.
Program Code
Program for resetting EEPROM (Upload this first):
//------------------Program Developed by R.GIRISH------------------//
#include<EEPROM.h>
int address = -1;
int value = 0;
int i = 0;
int j = 0;
int k = 0;
void setup()
{
Serial.begin(9600);
Serial.println("Reading EEPROM:");
for(i = 0; i<10; i++)
{
Serial.println(EEPROM.read(i));
}
Serial.println("Wrting null value:");
for(j = 0; j<10; j++)
{
address = address + 1;
EEPROM.write(address, value);
}
for(k = 0; k<10; k++)
{
Serial.println(EEPROM.read(i));
}
Serial.println("Done!!!");
}
void loop()
{
// Do nothing here.
}
//------------------Program Developed by R.GIRISH------------------//
Main Program (Upload this second):
//------------------Program Developed by R.GIRISH------------------//
#include <Keypad.h>
#include<EEPROM.h>
#include<LiquidCrystal.h>
LiquidCrystal lcd(A5, A4, A3, A2, A1, A0);
const int relay = 10;
const int LED = 11;
const byte ROWS = 4;
const byte COLS = 4;
char key1;
char key2;
char key3;
char key4;
char key5;
char key6;
char keyABCD;
char compkey1;
char compkey2;
char compkey3;
char compkey4;
char compkey5;
char compkey6;
int wordAddress1 = 0;
int wordAddress2 = 1;
int wordAddress3 = 2;
int wordAddress4 = 3;
int wordAddress5 = 4;
int wordAddress6 = 5;
int outputStatusAddress = 6;
int outputStatusValue = 0;
int passwordExistAddress = 7;
int passwordExistValue = 0;
int toggleAddress = 8;
int toggleValue = 0;
int check = 0;
char keys[ROWS][COLS] =
{
{'D','#','0','*'},
{'C','9','8','7'},
{'B','6','5','4'},
{'A','3','2','1'}
};
byte rowPins[ROWS] = {6,7,8,9};
byte colPins[COLS] = {2,3,4,5};
Keypad keypad = Keypad( makeKeymap(keys), rowPins, colPins, ROWS, COLS );
void setup()
{
lcd.begin(16,2);
pinMode(relay, OUTPUT);
digitalWrite(relay, LOW);
pinMode(LED, OUTPUT);
digitalWrite(LED, LOW);
lcd.clear();
lcd.setCursor(0,0);
lcd.print(" WELCOME");
lcd.setCursor(0,1);
lcd.print("****************");
delay(2000);
}
void loop()
{
apex:
passwordExistValue = EEPROM.read(passwordExistAddress);
if(passwordExistValue == 1) {goto normal;}
if(passwordExistValue != 1)
{
top:
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Set new 6 digit");
lcd.setCursor(0,1);
lcd.print("pin numer:");
key1 = keypad.waitForKey();
if((key1 == '*') || (key1 == '#') || (key1 == 'A') || (key1 == 'B') || (key1 == 'C') || (key1 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto top;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("*");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
key2 = keypad.waitForKey();
if((key2 == '*') || (key2 == '#') || (key2 == 'A') || (key2 == 'B') || (key2 == 'C') || (key2 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto top;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("**");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
key3 = keypad.waitForKey();
if((key3 == '*') || (key3 == '#') || (key3 == 'A') || (key3 == 'B') || (key3 == 'C') || (key3 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto top;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("***");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
key4 = keypad.waitForKey();
if((key4 == '*') || (key4 == '#') || (key4 == 'A') || (key4 == 'B') || (key4 == 'C') || (key4 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto top;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("****");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
key5 = keypad.waitForKey();
if((key5 == '*') || (key5 == '#') || (key5 == 'A') || (key5 == 'B') || (key5 == 'C') || (key5 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto top;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("*****");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
key6 = keypad.waitForKey();
if((key6 == '*') || (key6 == '#') || (key6 == 'A') || (key6 == 'B') || (key6 == 'C') || (key6 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto top;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("******");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
}
keyABCD = keypad.waitForKey();
if(keyABCD == 'A')
{
wrong:
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Re-type PIN NO:");
lcd.setCursor(0,1);
lcd.print("----------------");
compkey1 = keypad.waitForKey();
if((compkey1 == '*') || (compkey1 == '#') || (compkey1 == 'A') || (compkey1 == 'B') || (compkey1 == 'C') || (compkey1 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto wrong;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("*");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
compkey2 = keypad.waitForKey();
if((compkey2 == '*') || (compkey2 == '#') || (compkey2 == 'A') || (compkey2 == 'B') || (compkey2 == 'C') || (compkey2 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto wrong;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("**");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
compkey3 = keypad.waitForKey();
if((compkey3 == '*') || (compkey3 == '#') || (compkey3 == 'A') || (compkey3 == 'B') || (compkey3 == 'C') || (compkey3 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto wrong;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("***");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
compkey4 = keypad.waitForKey();
if((compkey4 == '*') || (compkey4 == '#') || (compkey4 == 'A') || (compkey4 == 'B') || (compkey4 == 'C') || (compkey4 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto wrong;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("****");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
compkey5 = keypad.waitForKey();
if((compkey5 == '*') || (compkey5 == '#') || (compkey5 == 'A') || (compkey5 == 'B') || (compkey5 == 'C') || (compkey5 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto wrong;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("*****");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
compkey6 = keypad.waitForKey();
if((compkey6 == '*') || (compkey6 == '#') || (compkey6 == 'A') || (compkey6 == 'B') || (compkey6 == 'C') || (compkey6 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto wrong;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("******");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
keyABCD = keypad.waitForKey();
if(keyABCD == 'A')
{
if(key1 == compkey1)
{
check = check + 1;
}
if(key2 == compkey2)
{
check = check + 1;
}
if(key3 == compkey3)
{
check = check + 1;
}
if(key4 == compkey4)
{
check = check + 1;
}
if(key5 == compkey5)
{
check = check + 1;
}
if(key6 == compkey6)
{
check = check + 1;
}
if(check == 6)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Password has");
lcd.setCursor(0,1);
lcd.print("been saved.");
delay(2000);
passwordExistValue = 1;
EEPROM.write(passwordExistAddress,passwordExistValue);
EEPROM.write(wordAddress1, key1);
EEPROM.write(wordAddress2, key2);
EEPROM.write(wordAddress3, key3);
EEPROM.write(wordAddress4, key4);
EEPROM.write(wordAddress5, key5);
EEPROM.write(wordAddress6, key6);
if(EEPROM.read(outputStatusAddress) == 1)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("STATUS:");
lcd.print("MAINS ON");
lcd.setCursor(0,1);
lcd.print("----------------");
digitalWrite(LED, HIGH);
digitalWrite(relay, HIGH);
toggleValue = 0;
EEPROM.write(toggleAddress,toggleValue);
}
if(EEPROM.read(outputStatusAddress) == 0)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("STATUS:");
lcd.print("MAINS OFF");
lcd.setCursor(0,1);
lcd.print("----------------");
digitalWrite(LED, LOW);
digitalWrite(relay, LOW);
toggleValue = 1;
EEPROM.write(toggleAddress,toggleValue);
}
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Password");
lcd.setCursor(0,1);
lcd.print("Mismatched !!!");
delay(2000);
goto top;
}
if(keyABCD == 'B')
{
goto wrong;
}
}
}
if(keyABCD == 'B')
{
goto top;
}
normal:
lcd.clear();
lcd.setCursor(0,0);
lcd.print("STATUS:");
if(EEPROM.read(outputStatusAddress) == 1)
{
lcd.print("MAINS ON");
lcd.setCursor(0,1);
lcd.print("----------------");
digitalWrite(LED, HIGH);
digitalWrite(relay, HIGH);
toggleValue = 0;
EEPROM.write(toggleAddress,toggleValue);
}
if(EEPROM.read(outputStatusAddress) == 0)
{
lcd.print("MAINS OFF");
lcd.setCursor(0,1);
lcd.print("----------------");
digitalWrite(LED, LOW);
digitalWrite(relay, LOW);
toggleValue = 1;
EEPROM.write(toggleAddress,toggleValue);
}
keyABCD = keypad.waitForKey();
if(keyABCD == 'C')
{
pass:
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter current");
lcd.setCursor(0,1);
lcd.print("PIN:");
key1 = keypad.waitForKey();
if((key1 == '*') || (key1 == '#') || (key1 == 'A') || (key1 == 'B') || (key1 == 'C') || (key1 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto pass;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("*");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
key2 = keypad.waitForKey();
if((key2 == '*') || (key2 == '#') || (key2 == 'A') || (key2 == 'B') || (key2 == 'C') || (key2 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto pass;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("**");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
key3 = keypad.waitForKey();
if((key3 == '*') || (key3 == '#') || (key3 == 'A') || (key3 == 'B') || (key3 == 'C') || (key3 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto pass;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("***");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
key4 = keypad.waitForKey();
if((key4 == '*') || (key4 == '#') || (key4 == 'A') || (key4 == 'B') || (key4 == 'C') || (key4 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto pass;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("****");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
key5 = keypad.waitForKey();
if((key5 == '*') || (key5 == '#') || (key5 == 'A') || (key5 == 'B') || (key5 == 'C') || (key5 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto pass;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("*****");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
key6 = keypad.waitForKey();
if((key6 == '*') || (key6 == '#') || (key6 == 'A') || (key6 == 'B') || (key6 == 'C') || (key6 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto pass;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("******");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
keyABCD = keypad.waitForKey();
if(keyABCD == 'A')
{
check = 0;
char readkey1 = EEPROM.read(wordAddress1);
char readkey2 = EEPROM.read(wordAddress2);
char readkey3 = EEPROM.read(wordAddress3);
char readkey4 = EEPROM.read(wordAddress4);
char readkey5 = EEPROM.read(wordAddress5);
char readkey6 = EEPROM.read(wordAddress6);
if(key1 == readkey1) {check = check + 1;}
if(key2 == readkey2) {check = check + 1;}
if(key3 == readkey3) {check = check + 1;}
if(key4 == readkey4) {check = check + 1;}
if(key5 == readkey5) {check = check + 1;}
if(key6 == readkey6) {check = check + 1;}
if(check == 6)
{
passwordExistValue = 0;
EEPROM.write(passwordExistAddress,passwordExistValue);
check = 0;
goto apex;
}
else if(check != 6)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Incorrect");
lcd.setCursor(0,1);
lcd.print("Pasword !!!");
delay(2000);
goto normal;
}
if(keyABCD == 'B') {goto normal;}
}
}
if(keyABCD == 'D')
{
toggle:
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter the PIN:");
lcd.setCursor(0,1);
key1 = keypad.waitForKey();
if((key1 == '*') || (key1 == '#') || (key1 == 'A') || (key1 == 'B') || (key1 == 'C') || (key1 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto toggle;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("*");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
key2 = keypad.waitForKey();
if((key2 == '*') || (key2 == '#') || (key2 == 'A') || (key2 == 'B') || (key2 == 'C') || (key2 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto toggle;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("**");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
key3 = keypad.waitForKey();
if((key3 == '*') || (key3 == '#') || (key3 == 'A') || (key3 == 'B') || (key3 == 'C') || (key3 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto toggle;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("***");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
key4 = keypad.waitForKey();
if((key4 == '*') || (key4 == '#') || (key4 == 'A') || (key4 == 'B') || (key4 == 'C') || (key4 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto toggle;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("****");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
key5 = keypad.waitForKey();
if((key5 == '*') || (key5 == '#') || (key5 == 'A') || (key5 == 'B') || (key5 == 'C') || (key5 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto toggle;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("*****");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
key6 = keypad.waitForKey();
if((key6 == '*') || (key6 == '#') || (key6 == 'A') || (key6 == 'B') || (key6 == 'C') || (key6 == 'D'))
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Enter 6 digit,");
lcd.setCursor(0,1);
lcd.print("then press 'A/B'.");
delay(2500);
goto toggle;
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("******");
lcd.setCursor(0,1);
lcd.print("YES 'A' NO 'B'");
}
check = 0;
if(EEPROM.read(wordAddress1) == key1)
{
check = check + 1;
}
if(EEPROM.read(wordAddress2) == key2)
{
check = check + 1;
}
if(EEPROM.read(wordAddress3) == key3)
{
check = check + 1;
}
if(EEPROM.read(wordAddress4) == key4)
{
check = check + 1;
}
if(EEPROM.read(wordAddress5) == key5)
{
check = check + 1;
}
if(EEPROM.read(wordAddress6) == key6)
{
check = check + 1;
}
keyABCD = keypad.waitForKey();
if(keyABCD == 'B')
{
goto normal;
}
if(keyABCD == 'A')
{
if(check == 6 && EEPROM.read(toggleAddress) == 1)
{
outputStatusValue = 1;
EEPROM.write(outputStatusAddress, outputStatusValue);
goto normal;
}
if(check == 6 && EEPROM.read(toggleAddress) == 0)
{
outputStatusValue = 0;
EEPROM.write(outputStatusAddress, outputStatusValue);
goto normal;
}
if(check != 6)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Incorrect");
lcd.setCursor(0,1);
lcd.print("Password !!!");
delay(1500);
goto normal;
}
}
}
}
//------------------Program Developed by R.GIRISH------------------//
phew!!….That’s a massive program code.
How to operate this password based mains ON/OFF switch project:
· With completed hardware setup, upload the EEPROM reset code.
· Now, upload the main program code.
· It will ask you to create 6-digit number password (no less or no more) on LCD, create a password and press “A”.
· Re-type the password again and press “A”. Your password is saved.
· You can change password by pressing the “C”. Enter the current password and enter the new password.
· To toggle the AC mains ON or OFF, press “D” and enter the password and press “A”.
Functions of keys A, B, C and D:
A – Enter/Yes
B – Cancel/NO
C – Change Password
D – Toggle AC Mains
Author’s Prototype:
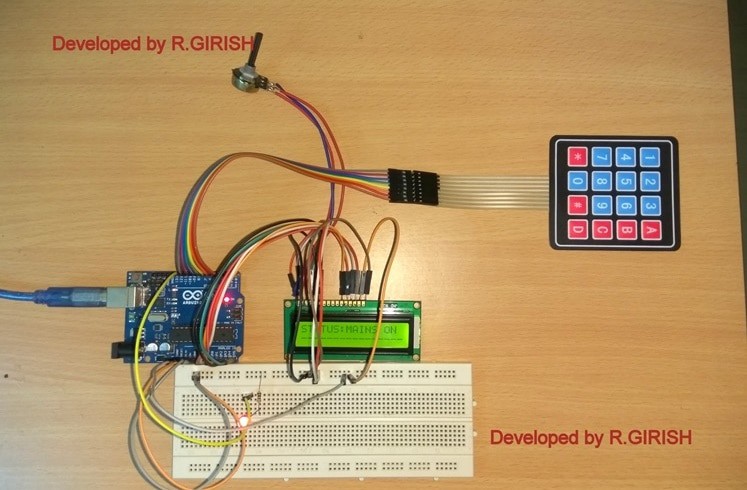
If you have any specific question regarding this password based AC Mains ON/OFF switch circuit project, express in the command section, you may receive a quick reply.
Have Questions? Please Comment below to Solve your Queries! Comments must be Related to the above Topic!!