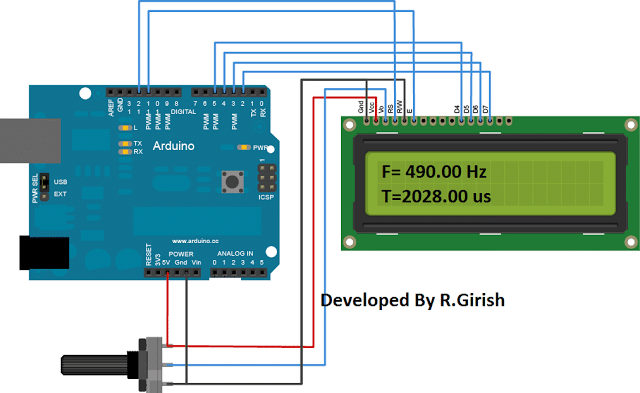
In this interesting post, we are going to make a simple single channel oscilloscope using Arduino and a personal computer, where the waveforms will be showcased on the PC’s display and frequency and time period of the input waves will be displayed on the 16 x 2 display.
Introduction
Every electronics enthusiast once said “I have a dream, one day I will purchase an oscilloscope” but, it is still dream of many to own a decent oscilloscope for their projects and experiments.
The oscilloscope being expensive equipment even for an entry level model, we consider them as a luxury electronics tool and we might bring our experiments and projects to halt because we can’t afford one.
This project might be a game changer for many, electronics enthusiasts no need to spend tons of money for an oscilloscope to measure basic parameters of a wave.
The proposed idea has very limited functionality so don’t expect the features on a high end oscilloscope to be present in this project. We get three solid functionalities from this project:
1) visual representation of waveform on computer’s screen
2) frequency measurement of the input wave
3) Time period measurement of input wave in microseconds.
The frequency and time period of the signal will be showcased on 16 x 2 LCD display. There are two methods for visually representing the waveform on the computer screen which will be described in later part of the article.
Now let’s dive into technical part of the setup.
The proposed setup consists of arduino which is the brain our project as usual, a 16 x 2 LCD display, IC 7404, 10K potentiometer and a computer preferably a windows machine.
The arduino is brain of the setup and we must choose Arduino UNO or Arduino mega or Arduino nano for this project since other models doesn’t have built-in USB to serial converter which is essential for communicating between Arduino and computer.
If we choose other models of arduino board we need external USB to serial converter which might complicate the project.
Illustration of LCD to Arduino connection:
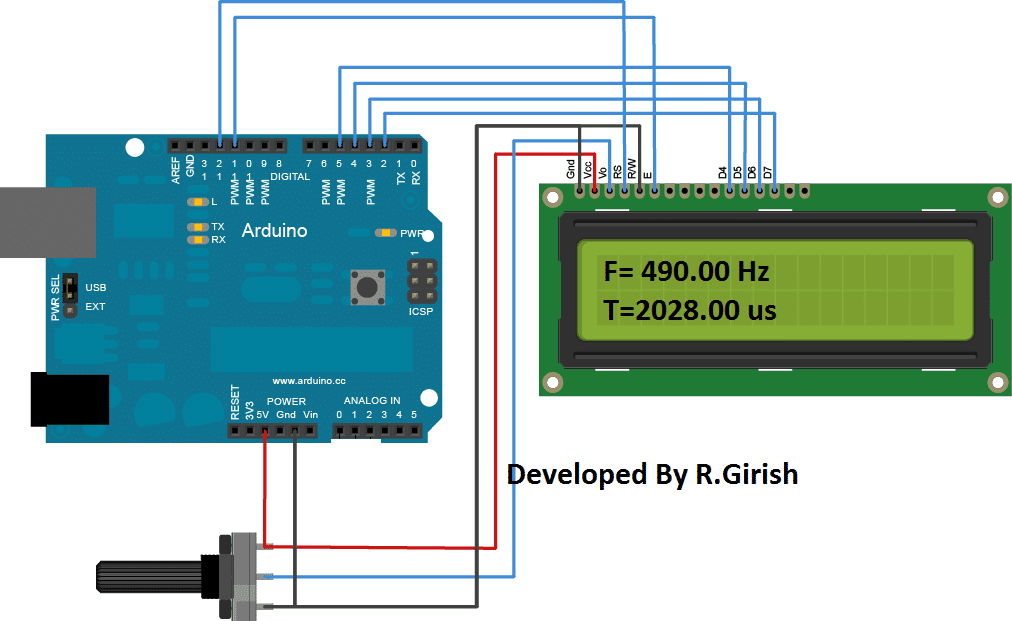
The above circuit is self-explanatory. We can find similar connection between the display and arduino on other LCD based projects.
The 10K potentiometer is used to adjust the contrast of the 16 x 2 LCD display which must be set by the user for optimum view.
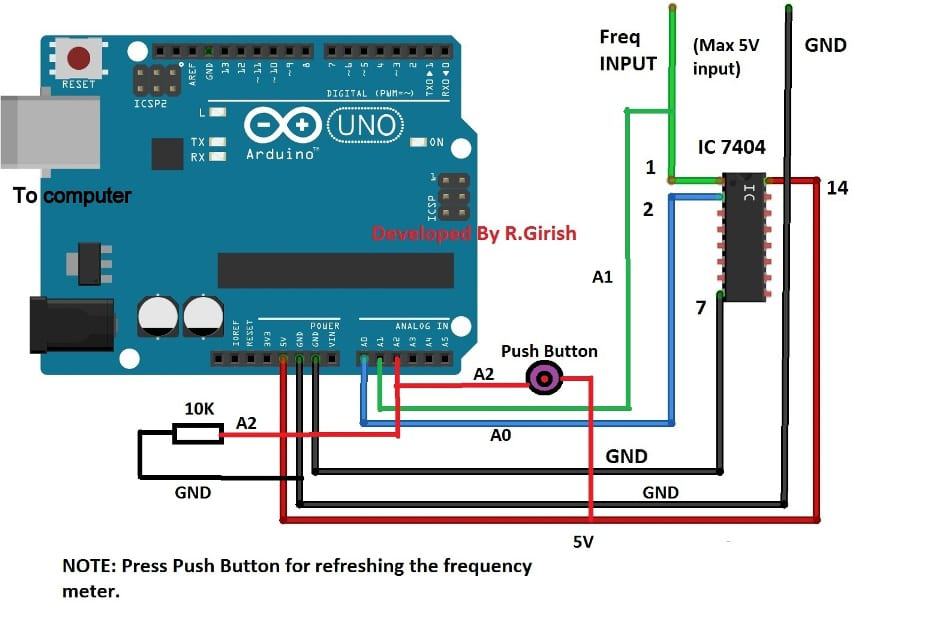
The function of IC 7404 is to eliminate any noise signal from the input and fed to frequency sampling pin A0. The IC 7404 only outputs rectangular waves which is a great advantage for arduino, since arduino is more capable of processing digital signal than analogue signals.
Program:
//-----Program Developed by R.Girish-----//
#include <LiquidCrystal.h>
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
int X;
int Y;
float Time;
float frequency;
const int Freqinput = A0;
const int oscInput = A1;
int Switch = A2;
const int test = 9;
void setup()
{
Serial.begin(9600);
lcd.begin(16,2);
pinMode(Switch,INPUT);
pinMode(Freqinput,INPUT);
pinMode(oscInput,INPUT);
pinMode(test, OUTPUT);
analogWrite(test,127);
lcd.setCursor(0,0);
lcd.print("Press the button");
}
void loop()
{
if(digitalRead(Switch)==HIGH)
{
lcd.clear();
lcd.setCursor(0,0);
X = pulseIn(Freqinput,HIGH);
Y = pulseIn(Freqinput,LOW);
Time = X+Y;
frequency = 1000000/Time;
if(frequency<=0)
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("F=");
lcd.print("0.00 Hz");
lcd.setCursor(0,1);
lcd.print("T=");
lcd.print("0.00 us");
}
else
{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("F=");
lcd.print(frequency);
lcd.print("Hz");
lcd.setCursor(0,1);
lcd.print("T=");
lcd.print(Time);
lcd.print(" us");
delay(500);
}
}
else
{
Serial.println(analogRead(oscInput));
}
}
//-----Program Developed by R.Girish-----//
Once you completed the hardware part and uploaded the above code. It’s time to plot waveform on the computer screen. This can done in two ways, the easiest and laziest way is described below.
Method 1:
• Connect the input wire to pin #9 of arduino (Test mode).
• Open the Arduino IDE (it must be 1.6.6 or above versions)
• Go to “tools” tab and select serial plotter
As soon as the serial plotter opens you can see the rectangular wave which is generated from arduino’s pin #9, illustrated below.
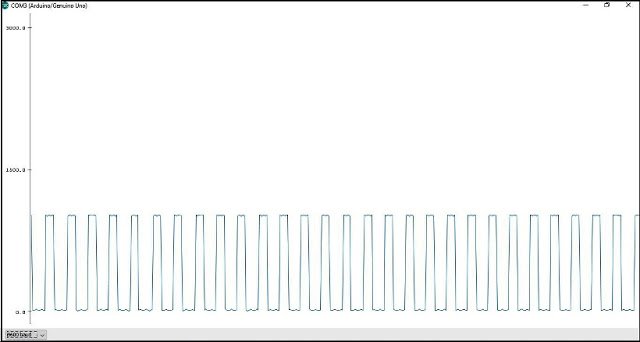
Press the push button to show the readings and also for refreshing the readings the LCD display, it must show around 490Hz on “test mode”.
Schematic of test mode:
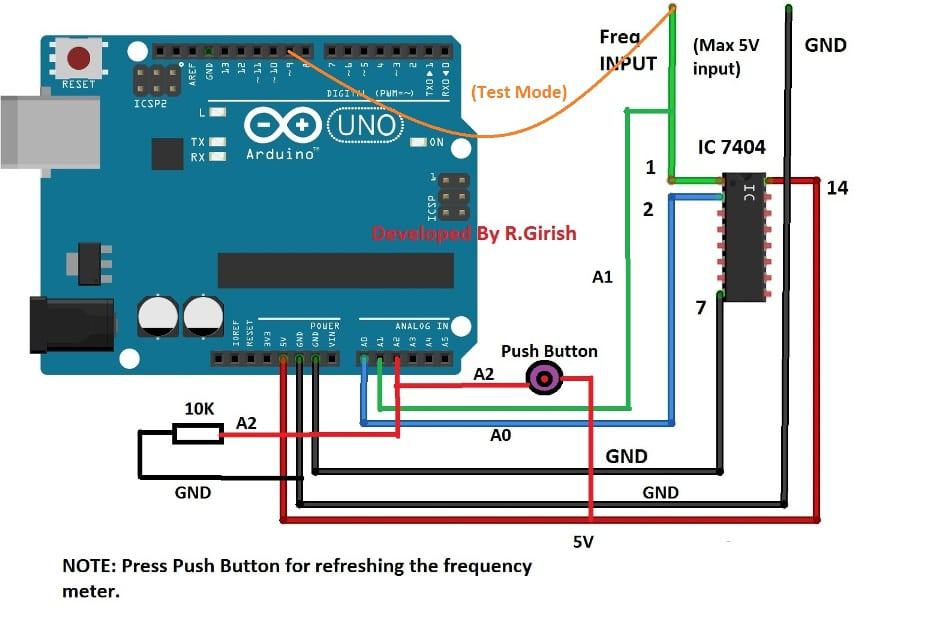
The test mode is to check proper functioning of the oscilloscope. The pin #9 is programed to give 490Hz output.
Method 2:
This method is relatively easy but we need to download software from the given link:
http://www.x-io.co.uk/downloads/Serial-Oscilloscope-v1.5.zip
This software will give us little more control and features compare to arduino’s serial plotter. We can zoom in and out of the generated waveform; we can set trigger functionality, offset control over vertical and horizontal axis etc.
• Download the software and extract.
• Now double click on the Serial Oscilloscope application.
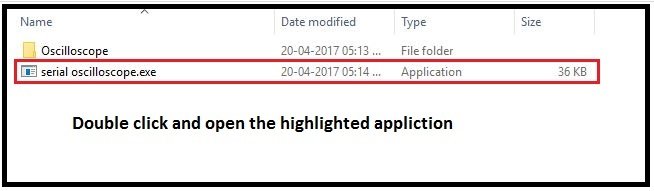
• A window will pop-up as illustrated below and select baud rate to 9600.
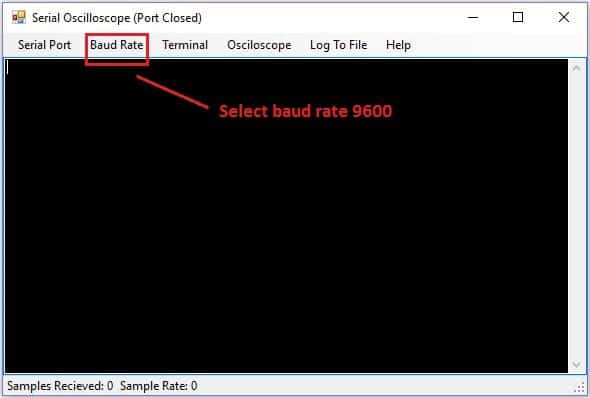
• Now select “Serial port” tab and select the right COM port which can vary computer to computer. If you select the correct COM port, you can see readings as illustrated below.
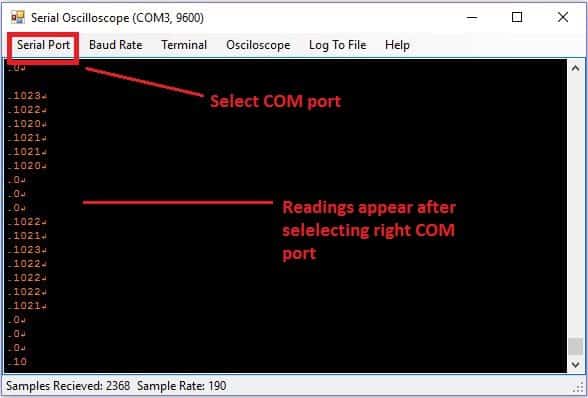
• Now select “oscilloscope” tab and select “channels 1, 2 and 3” (first option).
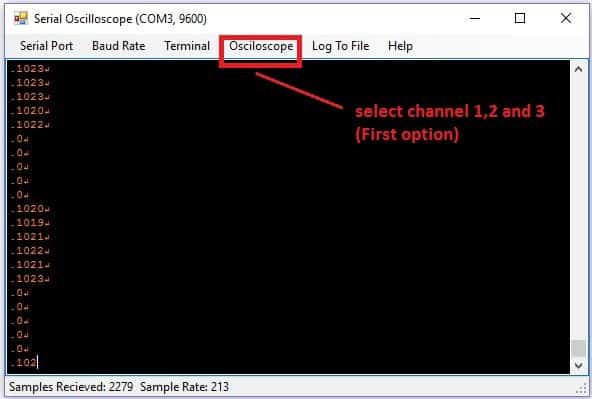
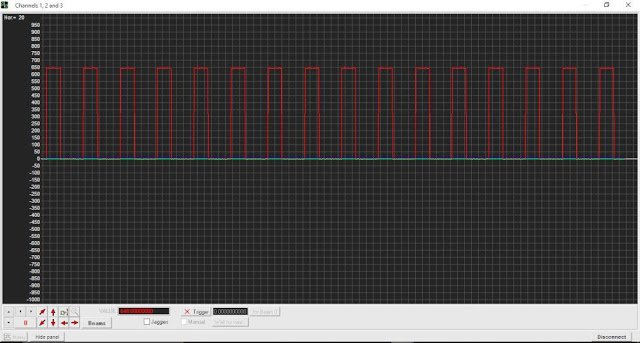
• You can see the generated test signal from Arduino as illustrated below.
As you can see there are some control buttons on the software by which you can analyze the waveform better.
NOTE:
The proposed setup has one major disadvantage:
The Arduino cannot show input waveform on computer screen and frequency/time period reading on LCD display simultaneously. To overcome this issue a push button is provided for reading/refresh the frequency and time period on the LCD display.
Once you press the button it will show the frequency and time period on LCD display at the same time waveform will freeze on the computer screen as long as you keep pressing the push button.
You may also consider this as an advantage since you can stop the frequency on the computer monitor at any instant and this may give you time to analyze displayed waveform.
Author’s prototype:
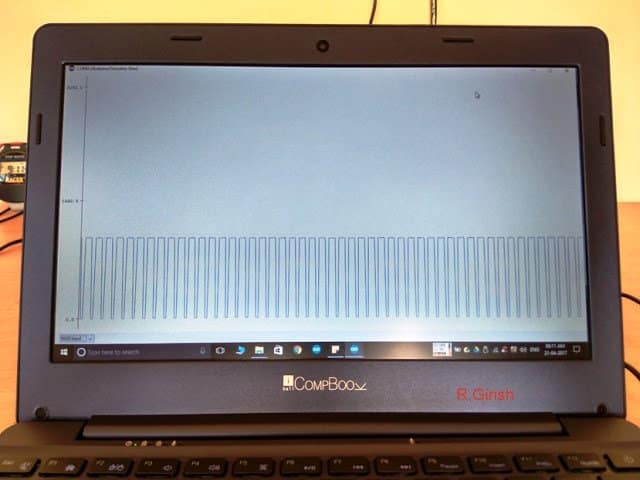
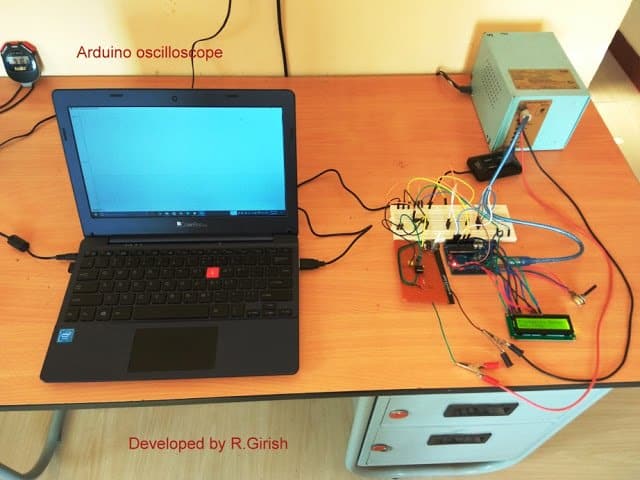
If you have any further queries regarding this simple single channel Arduino oscilloscope circuit, please feel free to use the below comment box for expressing your specific views
With over 50,000 comments answered so far, this is the only electronics website dedicated to solving all your circuit-related problems. If you’re stuck on a circuit, please leave your question in the comment box, and I will try to solve it ASAP!